EN
React - use Google Ads / GPT
6 points
In this short article, we would like to show how to use Google Ads / GPT in React.
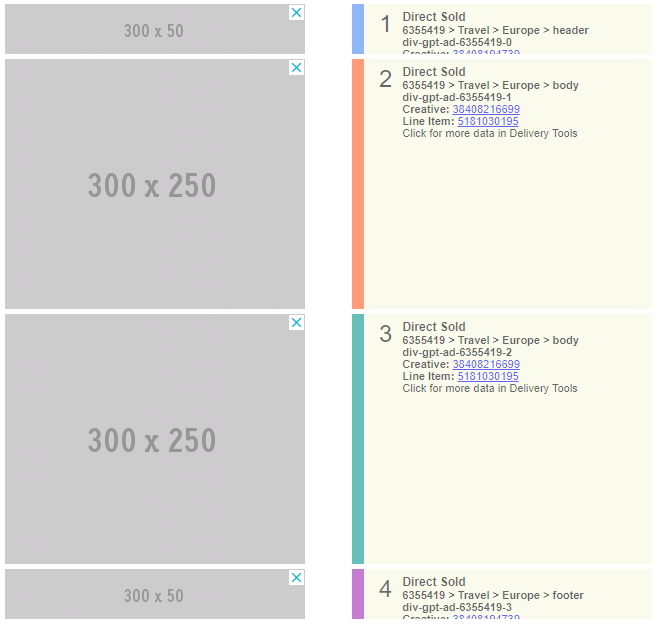
Note: read official documentation to know more about GPT.
Hints:
Practical example:
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
6
div {
7
margin: 5px;
8
}
9
10
</style>
11
12
<!-- Required only to run React code in this example -->
13
<script src="https://unpkg.com/react/umd/react.development.js"></script>
14
<script src="https://unpkg.com/react-dom/umd/react-dom.development.js"></script>
15
<script src="https://unpkg.com/@babel/standalone/babel.min.js"></script>
16
17
<!-- Google Ads / GPT import - attach it to yours project -->
18
<script src="https://www.googletagservices.com/tag/js/gpt.js"></script>
19
20
</head>
21
<body>
22
<div id="root"></div>
23
<!-- copy below script body to React project -->
24
<script type="text/babel">
25
26
//Note: Uncomment import lines during working with JSX Compiler.
27
28
// import React from 'react';
29
// import ReactDOM from 'react-dom';
30
31
const googletag = window.googletag || (window.googletag = { cmd: [] });
32
33
const createScope = (action) => action && action();
34
35
const GPTAdsManager = createScope(() => {
36
let initialized = false;
37
const initializeAds = (initialLoading = false, singleRequest = true) => {
38
if (initialized) {
39
return;
40
}
41
initialized = true;
42
googletag.cmd.push(() => {
43
const pubads = googletag.pubads();
44
if (!initialLoading) {
45
pubads.disableInitialLoad();
46
}
47
if (singleRequest) {
48
pubads.enableSingleRequest();
49
}
50
googletag.enableServices();
51
});
52
};
53
const createSlot = (adPath, adWidth, adHeight, elementId) => {
54
initializeAds(); // only if not initialized yet
55
let slot = null;
56
googletag.cmd.push(() => {
57
const size = adWidth & adHeight ? [adWidth, adHeight] : ['fluid'];
58
const tmp = googletag.defineSlot(adPath, size, elementId);
59
if (tmp) {
60
slot = tmp;
61
tmp.addService(googletag.pubads());
62
}
63
});
64
const display = () => {
65
if (slot) {
66
googletag.cmd.push(() => {
67
const pubads = googletag.pubads();
68
pubads.refresh([slot]);
69
});
70
}
71
};
72
const refresh = () => {
73
if (slot) {
74
googletag.cmd.push(() => {
75
const pubads = googletag.pubads();
76
pubads.refresh([slot]);
77
});
78
}
79
};
80
const destroy = () => {
81
if (slot) {
82
const tmp = slot;
83
googletag.cmd.push(() => {
84
const pubads = googletag.pubads();
85
googletag.destroySlots([tmp]);
86
});
87
slot = null;
88
}
89
};
90
return { display, refresh, destroy };
91
}
92
return { initializeAds, createSlot };
93
});
94
95
let adCounter = 0;
96
97
const Ad = ({ path, width, height }) => {
98
const id = React.useMemo(() => `div-gpt-ad-${++adCounter}`, []);
99
React.useEffect(() => {
100
const slot = GPTAdsManager.createSlot(path, width, height, id);
101
slot.display();
102
// slot.refresh(); // forces Ad reloading
103
return () => {
104
slot.destroy();
105
};
106
}, [path, width, height]);
107
return (
108
<div id={id} />
109
);
110
};
111
112
// Usage example:
113
114
// somewhere in the code...
115
GPTAdsManager.initializeAds(false, true);
116
117
// uncomment below 3 lines to open GPT Ads console
118
// window.googletag.cmd.push(() => {
119
// window.googletag.openConsole();
120
// });
121
122
const App = () => (
123
<div className="App">
124
<Ad path="/6355419/Travel/Europe/header" width={300} height={50} />
125
<Ad path="/6355419/Travel/Europe/body" width={300} height={250} />
126
<Ad path="/6355419/Travel/Europe/body" width={300} height={250} />
127
<Ad path="/6355419/Travel/Europe/footer" width={300} height={50} />
128
</div>
129
);
130
131
const root = document.querySelector('#root');
132
ReactDOM.render(<App />, root );
133
134
</script>
135
</body>
136
</html>
Note: be sure that Ad size has always the same size to prevent unnecessary Ad recreation - or change it consciously according to new slot configurations.