EN
React - simple page layout
6 points
In this short article we would like to show you how to make simple web page template in React.
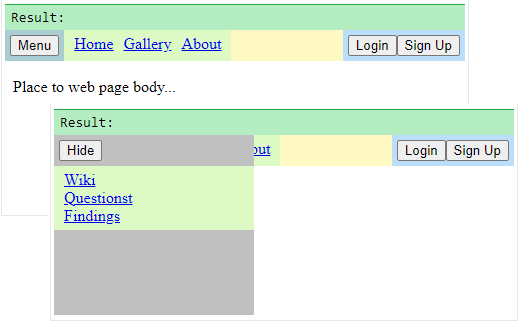
Practical example:
xxxxxxxxxx
1
// Note: Uncomment import lines during working with JSX Compiler.
2
// import React from 'react';
3
// import ReactDOM from 'react-dom';
4
5
const linksStyle = {
6
padding: '5px',
7
background: '#ddf9c4',
8
flex: 'none'
9
};
10
11
const linkStyle = {
12
padding: '0 5px'
13
};
14
15
const spacerStyle = {
16
background: '#fff9c4',
17
flex: '1'
18
};
19
20
// Navbar -------------------------------------
21
22
const navbarStyles = {
23
navbar: {
24
position: 'fixed',
25
top: '0',
26
left: '0',
27
right: '0',
28
display: 'flex',
29
zIndex: '900',
30
siteMenu: {
31
padding: '5px',
32
background: '#aacdd2',
33
flex: 'none'
34
},
35
userMenu: {
36
padding: '5px',
37
background: '#bbdefb',
38
flex: 'none'
39
}
40
}
41
};
42
43
const Navbar = ({onSiteMenuShow}) => {
44
return (
45
<div style={navbarStyles.navbar}>
46
<div style={navbarStyles.navbar.siteMenu}>
47
<button onClick={onSiteMenuShow}>Menu</button>
48
</div>
49
<div style={linksStyle}>
50
<a style={linkStyle} href='#'>Home</a>
51
<a style={linkStyle} href='#'>Gallery</a>
52
<a style={linkStyle} href='#'>About</a>
53
</div>
54
<div style={spacerStyle}></div>
55
<div style={navbarStyles.navbar.userMenu}>
56
<button>Login</button>
57
<button>Sign Up</button>
58
</div>
59
</div>
60
);
61
};
62
63
// Menu ------------------------------------
64
65
const siteMenuStyles = {
66
menu: {
67
position: 'fixed',
68
left: 0,
69
top: 0,
70
bottom: 0,
71
background: 'silver',
72
overflow: 'hidden',
73
transition: '0.3s',
74
zIndex: '1000',
75
header: {
76
padding: '5px'
77
}
78
}
79
};
80
81
siteMenuStyles.menu.visible = {
82
siteMenuStyles.menu,
83
width: '200px'
84
};
85
86
siteMenuStyles.menu.hidden = {
87
siteMenuStyles.menu,
88
width: '0px'
89
};
90
91
const SiteMenu = ({visible, onClose}) => {
92
return (
93
<div style={siteMenuStyles.menu[visible ? 'visible' : 'hidden']}>
94
<div style={siteMenuStyles.menu.header}>
95
<button onClick={onClose}>Hide</button>
96
</div>
97
<div>
98
<div style={linksStyle}>
99
<div><a style={linkStyle} href='#'>Wiki</a></div>
100
<div><a style={linkStyle} href='#'>Questionst</a></div>
101
<div><a style={linkStyle} href='#'>Findings</a></div>
102
</div>
103
</div>
104
</div>
105
);
106
};
107
108
// App -------------------------------------
109
110
const appStyle = {
111
padding: '40px 0 0 0',
112
height: '100px'
113
};
114
115
const App = () => {
116
const [menuVisible, setMenuVisible] = React.useState(false);
117
const handleSiteMenuShow = () => setMenuVisible(true);
118
const handleSiteMenuHide = () => setMenuVisible(false);
119
return (
120
<div style={appStyle}>
121
<Navbar onSiteMenuShow={handleSiteMenuShow} />
122
<SiteMenu visible={menuVisible} onClose={handleSiteMenuHide} />
123
<div>Place to web page body...</div>
124
</div>
125
);
126
};
127
128
const root = document.querySelector('#root');
129
ReactDOM.render(<App />, root);