EN
JavaScript - use multiple Google Ads / GPT
8
points
In this short article, we would like to show how to use multiple Google Ads / GPT in JavaScript.
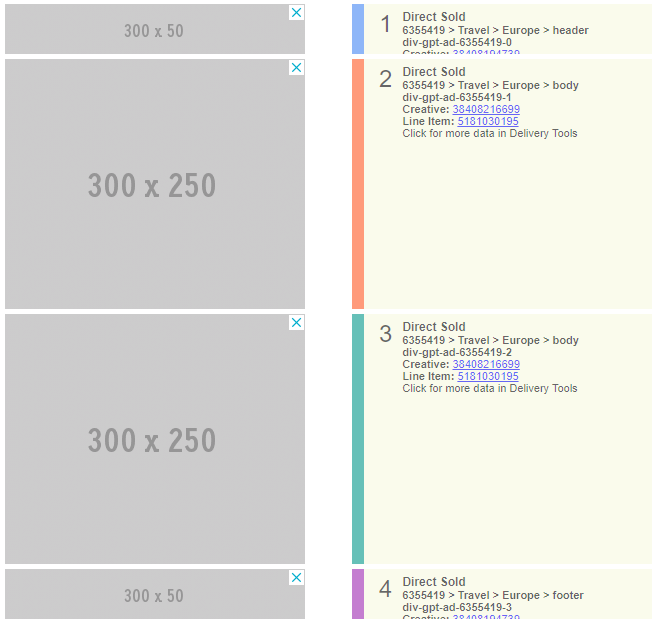
Note: read official documentation to know more about GPT.
Hints:
- run below code under your domain and use proper paths and sizes for slots to see the effect,
- if you are using AdBlock disable it - you can find AdBlock detection test here.
The below example displays 4 Ads (single slot displays a single ad):
slot1
in site header:
path:/6355419/Travel/Europe/header
with size:300x50
px,slot2
andslot3
display the same ad in the site body:
path:/6355419/Travel/Europe/body
with size:300x250
px,slot4
in site footer:
path:/6355419/Travel/Europe/footer
with size:300x50
px.
Note:
/6355419/Travel/Europe
was presented in the examples in the official documentation.
Practical example:
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<style>
div {
margin: 5px;
}
</style>
<script src="//www.googletagservices.com/tag/js/gpt.js"></script>
<!--
ALWAYS USE THE SAME PROTOCOL like your web page uses:
<script src="http://www.googletagservices.com/tag/js/gpt.js"></script>
<script src="https://www.googletagservices.com/tag/js/gpt.js"></script>
-->
<script type="text/javascript">
const googletag = window.googletag || (window.googletag = { cmd: [] });
const createScope = (action) => action && action();
const GPTAdsManager = createScope(() => {
let initialized = false;
const initializeAds = (initialLoading = false, singleRequest = true) => {
if (initialized) {
return;
}
initialized = true;
googletag.cmd.push(() => {
const pubads = googletag.pubads();
if (!initialLoading) {
pubads.disableInitialLoad();
}
if (singleRequest) {
pubads.enableSingleRequest();
}
googletag.enableServices();
});
};
const createSlot = (adPath, adWidth, adHeight, elementId) => {
initializeAds(); // only if not initialized yet
let slot = null;
googletag.cmd.push(() => {
const size = adWidth & adHeight ? [adWidth, adHeight] : ['fluid'];
const tmp = googletag.defineSlot(adPath, size, elementId);
if (tmp) {
slot = tmp;
tmp.addService(googletag.pubads());
}
});
const display = () => {
if (slot) {
googletag.cmd.push(() => {
const pubads = googletag.pubads();
pubads.refresh([slot]);
});
}
};
const refresh = () => {
if (slot) {
googletag.cmd.push(() => {
const pubads = googletag.pubads();
pubads.refresh([slot]);
});
}
};
const destroy = () => {
if (slot) {
const tmp = slot;
googletag.cmd.push(() => {
const pubads = googletag.pubads();
googletag.destroySlots([tmp]);
});
slot = null;
}
};
return { display, refresh, destroy };
}
return { initializeAds, createSlot };
});
</script>
</head>
<body>
<div class="header">
<div id='div-gpt-ad-6355419-0'></div>
</div>
<div class="body">
<div id='div-gpt-ad-6355419-1'></div>
<div id='div-gpt-ad-6355419-2'></div>
</div>
<div class="footer">
<div id='div-gpt-ad-6355419-3'></div>
</div>
<script type='text/javascript'>
GPTAdsManager.initializeAds(false, true); // initialLoading=false, singleRequest=true
// uncomment the below lines to open GPT Ads console
// googletag.cmd.push(() => {
// googletag.openConsole();
// });
const slot1 = GPTAdsManager.createSlot('/6355419/Travel/Europe/header', 300, 50, 'div-gpt-ad-6355419-0');
const slot2 = GPTAdsManager.createSlot('/6355419/Travel/Europe/body', 300, 250, 'div-gpt-ad-6355419-1');
const slot3 = GPTAdsManager.createSlot('/6355419/Travel/Europe/body', 300, 250, 'div-gpt-ad-6355419-2');
const slot4 = GPTAdsManager.createSlot('/6355419/Travel/Europe/footer', 300, 50, 'div-gpt-ad-6355419-3');
slot1.display(); // display ad in slot 1
slot2.display();
slot3.display();
slot4.display();
// slot1.refresh(); // refreshes ad in slot 1
// slot1.destroy(); // destroys ad in slot 1
</script>
</body>
</html>