EN
React - multiple files input
3 points
In this article, we would like to show you how to upload multiple files in React.
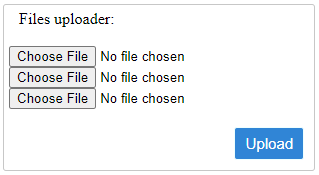
There are two main approaches how to let users to select multiple files:
- using
multiple
property withinput
element that allows to select in many files per oneinput
, - using multiple
input
elements that each oneinput
let's user to select only one file.
In this article, we want to show how to use the second option.
The below example uses multiple <input type="file" />
elements that help us to select files from a computer and upload them with fetch()
method that uses FormData
class object to wrap files like classic form
element submit action does, but here we send it via AJAX / XHR request. It is very important to give for each input
element proper name
to be able to distinct it on the server - the below example uses file1
, file2
and file3
.
Runnable example:
xxxxxxxxxx
1
// Note: Uncomment import lines during working with JSX Compiler.
2
// import React from 'react';
3
// import ReactDOM from 'react-dom';
4
5
const formStyle = {
6
margin: 10,
7
padding: 5,
8
border: '1px solid #c7c7c7',
9
borderRadius: 3,
10
width: 300
11
}
12
13
const submitStyle = {
14
margin: '0 5px 5px auto',
15
padding: '7px 10px',
16
border: '1px solid #efffff',
17
borderRadius: 3,
18
background: '#3085d6',
19
fontSize: 15,
20
color: 'white',
21
display: 'flex',
22
cursor: 'pointer'
23
}
24
25
const labelStyle = {
26
padding: 10,
27
border: 10
28
}
29
30
const Form = () => {
31
const handleSubmit = (e) => {
32
e.preventDefault();
33
const formData = new FormData(e.target);
34
fetch('/example/upload', { method: 'POST', body: formData })
35
.then(response => response.text()) // or response.json()
36
.then(responseText => {
37
console.log(responseText);
38
})
39
.catch(error => {
40
console.error('File upload error!');
41
});
42
};
43
return (
44
<form onSubmit={handleSubmit} style={formStyle}>
45
<label htmlFor="userfile" style={labelStyle}>Files uploader:</label>
46
<br />
47
<br />
48
<input type="file" name="file1" /><br />
49
<input type="file" name="file2" /><br />
50
<input type="file" name="file3" /><br />
51
<br />
52
<input style={submitStyle} type="submit" value="Upload" />
53
</form>
54
);
55
};
56
57
const root = document.querySelector('#root');
58
ReactDOM.render(<Form />, root);
Important:
- each file must be given a name,
- the number of occurrences of the
<input type = "file" />
element indicates the number of files that we send to send to the server.