EN
React - three-state checkbox (with indeterminate property)
12
points
In this short article, we would like to show you how to create three-state checkbox in React with input
checkbox
element that uses built-in indeterminate
property. Three-state checkbox we understand as checkbox that is able to get three states: true
, false
and null
as unset value.
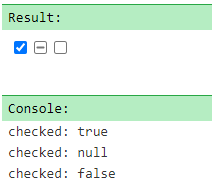
Practical example:
// ONLINE-RUNNER:browser;
// import React from 'react';
// import ReactDOM from 'react-dom';
const updateInput = (ref, checked) => {
const input = ref.current;
if (input) {
input.checked = checked;
input.indeterminate = checked == null;
}
};
const ThreeStateCheckbox = ({name, checked, onChange}) => {
const inputRef = React.useRef(null);
const checkedRef = React.useRef(checked);
React.useEffect(() => {
checkedRef.current = checked;
updateInput(inputRef, checked);
}, [checked]);
const handleClick = () => {
switch (checkedRef.current) {
case true:
checkedRef.current = false;
break;
case false:
checkedRef.current = null;
break;
default: // null
checkedRef.current = true;
break;
}
updateInput(inputRef, checkedRef.current);
if (onChange) {
onChange(checkedRef.current);
}
};
return (
<input ref={inputRef} type="checkbox" name={name} onClick={handleClick} />
);
};
const App = () => {
const [checked, setChecked] = React.useState(null);
const handleChange = (checked) => {
console.log(`checked: ${checked}`);
};
return (
<ThreeStateCheckbox checked={checked} onChange={handleChange} />
);
};
const root = document.querySelector('#root');
ReactDOM.render(<App />, root );