EN
React - async image loading
12
points
In this short article, we would like to show how to load images in an async mode in React.
Quick solution (add loading="lazy"
to img
elements):
<img src="https://dirask.com/static/bucket/1574890428058-BZOQxN2D3p--image.png" loading="lazy" />
As async mode we understand the images loading occurs after all resources are loaded, do not blocking other sync resources loading. That approach speeds up page loading by splitting the loading process into 2 steps:
- page loading (without async images) - we see all necessary things in the correct way faster,
- async images loading - images are loaded when the page is ready.
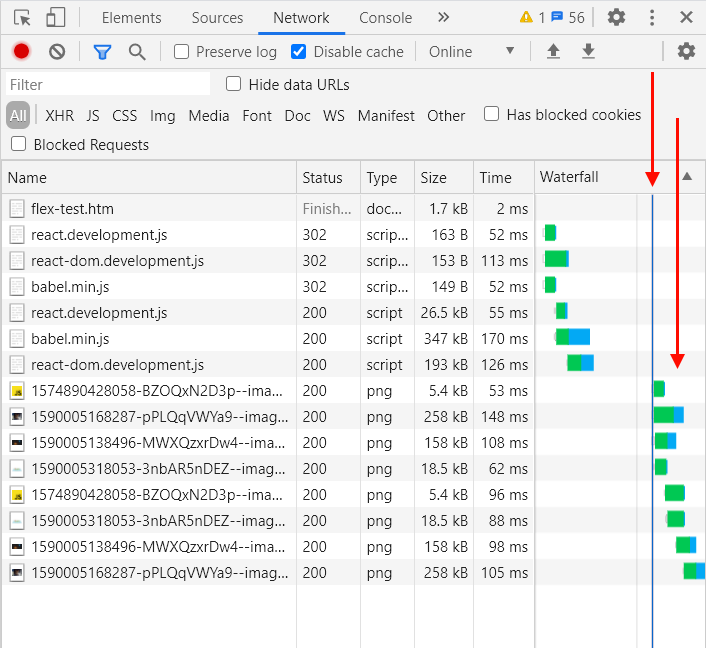
Practical example
The main advantage of this solution is the web browser decides when image should be loaded to achieve the best performance.
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<body>
<img src="https://dirask.com/static/bucket/1574890428058-BZOQxN2D3p--image.png" loading="lazy" />
<p>Some text here ...</p>
<img src="https://dirask.com/static/bucket/1590005168287-pPLQqVWYa9--image.png" loading="lazy" />
<p>Some text here ...</p>
<img src="https://dirask.com/static/bucket/1590005138496-MWXQzxrDw4--image.png" loading="lazy" />
<p>Some text here ...</p>
<img src="https://dirask.com/static/bucket/1590005318053-3nbAR5nDEZ--image.png" loading="lazy" />
</body>
</html>
Custom solution
In this section you can find solutuion that lets to load images in async mode, even the browser doesn't support loading="lazy"
.
// ONLINE-RUNNER:browser;
//import React from 'react';
//import ReactDOM from 'react-dom';
const AsyncImage = (props) => {
const [loadedSrc, setLoadedSrc] = React.useState(null);
React.useEffect(() => {
setLoadedSrc(null);
if (props.src) {
const handleLoad = () => {
setLoadedSrc(props.src);
};
const image = new Image();
image.addEventListener('load', handleLoad);
image.src = props.src;
return () => {
image.removeEventListener('load', handleLoad);
};
}
}, [props.src]);
if (loadedSrc === props.src) {
return (
<img {...props} />
);
}
return null;
};
const App = () => {
return (
<div>
<AsyncImage src="https://dirask.com/static/bucket/1574890428058-BZOQxN2D3p--image.png" />
<p>Some text here ...</p>
<AsyncImage src="https://dirask.com/static/bucket/1590005168287-pPLQqVWYa9--image.png" />
<p>Some text here ...</p>
<AsyncImage src="https://dirask.com/static/bucket/1590005138496-MWXQzxrDw4--image.png" />
<p>Some text here ...</p>
<AsyncImage src="https://dirask.com/static/bucket/1590005318053-3nbAR5nDEZ--image.png" />
</div>
);
};
const root = document.querySelector('#root');
ReactDOM.render(<App />, root);