PL
React - trójstanowe pole wyboru (checkbox)
6 points
W tym krótkim artykule chcielibyśmy pokazać, jak utworzyć trójstanowe pole wyboru w Reakcie - trzy-stanowy przycisk checkbox
.
W tym celu tworzony jest element input
typu checkbox
, który używa wbudowanej własności indeterminate
. Trójstanowe pole wyboru rozumiemy jako pole, które jest w stanie uzyskać trzy stany: true
, false
i null
. null
jest wartościę nieustawioną.
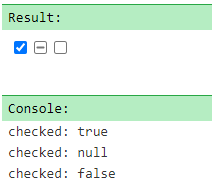
Praktyczny przykład:
xxxxxxxxxx
1
// Uwaga: Odkomentuj poniższe linijki podczas pracy z kompilatorem JSX:
2
// import React from 'react';
3
// import ReactDOM from 'react-dom';
4
5
const updateInput = (ref, checked) => {
6
const input = ref.current;
7
if (input) {
8
input.checked = checked;
9
input.indeterminate = checked == null;
10
}
11
};
12
13
const ThreeStateCheckbox = ({name, checked, onChange}) => {
14
const inputRef = React.useRef(null);
15
const checkedRef = React.useRef(checked);
16
React.useEffect(() => {
17
checkedRef.current = checked;
18
updateInput(inputRef, checked);
19
}, [checked]);
20
const handleClick = () => {
21
switch (checkedRef.current) {
22
case true:
23
checkedRef.current = false;
24
break;
25
case false:
26
checkedRef.current = null;
27
break;
28
default: // null
29
checkedRef.current = true;
30
break;
31
}
32
updateInput(inputRef, checkedRef.current);
33
if (onChange) {
34
onChange(checkedRef.current);
35
}
36
};
37
return (
38
<input ref={inputRef} type="checkbox" name={name} onClick={handleClick} />
39
);
40
};
41
42
const App = () => {
43
const [checked, setChecked] = React.useState(null);
44
const handleChange = (checked) => {
45
console.log(`checked: ${checked}`);
46
};
47
return (
48
<ThreeStateCheckbox checked={checked} onChange={handleChange} />
49
);
50
};
51
52
const root = document.querySelector('#root');
53
ReactDOM.render(<App />, root );