EN
React - multiple radio buttons
3
points
In this article we would like to show you how to use multiple radio buttons in React.
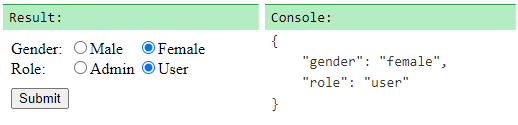
Below example uses RadioInput
functional component which renders label
with a single input type="radio"
(radio button).
In our Form
we have two groups of RadioInput
elements. One group for setting gender
, second for setting role
.
In every group we can select only one radio button at the same time, then in Gender group setGender
function sets gender
and in Role group setRole
function sets role
depends on which option we chose.
Practical example:
// ONLINE-RUNNER:browser;
//Note: Uncomment import lines during working with JSX Compiler.
// import React from 'react';
const RadioInput = ({label, value, checked, setter}) => {
return (
<label>
<input type="radio" checked={checked == value}
onChange={() => setter(value)} />
<span>{label}</span>
</label>
);
};
const Form = props => {
const [gender, setGender] = React.useState();
const [role, setRole] = React.useState();
const handleSubmit = e => {
e.preventDefault();
const data = {gender, role};
const json = JSON.stringify(data, null, 4);
console.clear();
console.log(json);
};
return (
<form onSubmit={handleSubmit}>
<div>
<label>Gender:</label>
<RadioInput label="Male" value="male" checked={gender} setter={setGender} />
<RadioInput label="Female" value="female" checked={gender} setter={setGender} />
</div>
<div>
<label>Role:</label>
<RadioInput label="Admin" value="admin" checked={role} setter={setRole} />
<RadioInput label="User" value="user" checked={role} setter={setRole} />
</div>
<button type="submit">Submit</button>
</form>
);
};
const root = document.querySelector('#root');
ReactDOM.render(<Form />, root );