Simple way to use multiple radio buttons in React
Many beginners haveĀ a problem with using multiple radio buttonsĀ because they do not realize that the radio buttons are grouped and only oneĀ radio button can be selected in oneĀ group.
Today, I'd like to show you how to easily use multiple radio buttons without using grouping by the input
name
property in React. 😊
Final effect:
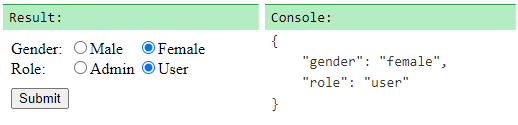
In below example I've createdĀ RadioInput
Ā functional component which rendersĀ label
Ā with a singleĀ input type="radio"
Ā (radio button).
In theĀ Form
Ā we have four RadioInput
Ā elements - two for gender and, two for role.
In every group we can selectĀ only one radio button atĀ the same time, thenĀ setGender
Ā function setsĀ genderĀ 🧒🧑Ā andĀ setRole
Ā function setsĀ roleĀ dependingĀ on which option we choose.Ā
Runnable example:
// ONLINE-RUNNER:browser;
/// Note: Uncomment import lines in your project.
// import React from 'react';
const RadioInput = ({label, value, checked, setter}) => {
return (
<label>
<input type="radio" checked={checked === value} onChange={() => setter(value)} />
<span>{label}</span>
</label>
);
};
const Form = (props) => {
const [gender, setGender] = React.useState();
const [role, setRole] = React.useState();
const handleSubmit = e => {
e.preventDefault();
const data = {gender, role};
const json = JSON.stringify(data, null, 4);
console.clear();
console.log(json);
};
return (
<form onSubmit={handleSubmit}>
<div>
<label>Gender:</label>
<RadioInput label="Male" value="male" checked={gender} setter={setGender} />
<RadioInput label="Female" value="female" checked={gender} setter={setGender} />
</div>
<div>
<label>Role:</label>
<RadioInput label="Admin" value="admin" checked={role} setter={setRole} />
<RadioInput label="User" value="user" checked={role} setter={setRole} />
</div>
<button type="submit">Submit</button>
</form>
);
};
const root = document.querySelector('#root');
ReactDOM.render(<Form />, root );
If you found this solution useful and would like to receive more content like this let me know by leavingĀ a reaction.
Thanks for your time and see you in the upcoming posts! 😊
Ā