EN
React - sweetalert example
3
points
In this article, we would like to show you how to create SweetAlert - a customizable replacement for JavaScript's popup boxes.
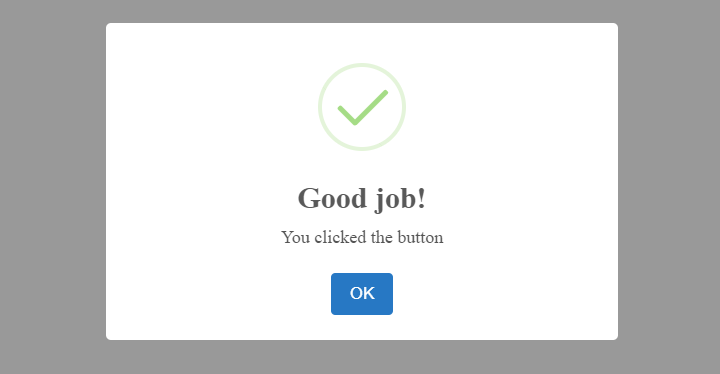
The first step is to install sweetalert2
package using npm:
$ npm install sweetalert2
Or grab from CDN:
<script src="//cdn.jsdelivr.net/npm/sweetalert2@10"></script>
Quick solution:
import Swal from 'sweetalert2'
Swal.fire('Alert text')
// or an example from the picture above
Swal.fire( 'Good job!','You clicked the button','success');
Below an example with different types of alerts:
- success alert,
- question alert,
- and alert with a custom image.
Note:
SweetAlert2
includes many more advanced options which you can check out by going here.
Practical example:
import React from 'react'
import Swal from 'sweetalert2'
const successAlert = () => {
Swal.fire({
title: 'Good job!',
text: 'You clicked the button.',
icon: 'success'
});
}
const questionAlert = () => {
Swal.fire({
title: 'Do you have a problem to solve?!',
text: 'Ask us on dirask',
icon: 'question'
});
}
const customImageAlert = () => {
Swal.fire({
text: "your custom image",
imageUrl: 'https://i.ibb.co/LktzszD/dirask.png'
});
}
const App = () => {
return (
<div>
<button onClick={successAlert}>Success Alert</button>
<button onClick={questionAlert}>Question Alert</button>
<button onClick={customImageAlert}>Custom image Alert</button>
</div>
);
}
export default App;
Online runnable example: