EN
React - render component as String (HTML in String)
4
points
In this article, we would like to show how to render component as HTML string in React.
Quick solution:
import React from 'react';
import ReactDOMServer from 'react-dom/server'
import App from './App';
const html = ReactDOMServer.renderToString(<App />);
// Where: html variable contains application rendered as HTML string.
Note: to know how to install
react-dom/server
check the below section.
Practical example
In this section you can see how using renderToString()
method, render application as HTML string, what is typically used in the server-side rendering (SSR).
Simple steps:
1. install react-dom/server
package using:
npm install react-dom
2. create index.jsx
file:
import React from 'react';
import ReactDOMServer from 'react-dom/server';
const App = () => {
return (
<div className="App">
Some text inside...
</div>
);
};
const html = ReactDOMServer.renderToString(<App/>);
console.log(html);
3. run *.js
file using:
node build/static/js/main.*.js
Result:
<div class="App" data-reactroot="">Some text inside...</div>
Screenshot:
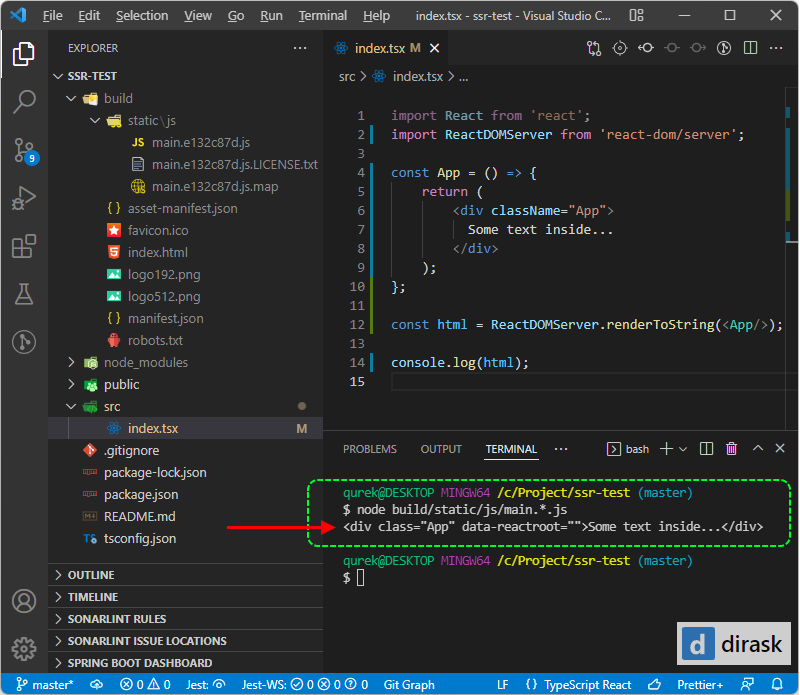