React - how to use environment variables
In this article, we would like to show you how to use environment variables in React.
While working with React, environment variables are variables that are available through a global process. Environment variables are embedded into the build, meaning anyone can view them by inspecting your app's files.
1. Defining environment variables in .env
file
To define environment variables you need to:
- Create new file in the root of your project and call it
.env
, - Inside
.env
file create a variable beginning with "REACT_APP_
" and assign it a value.
Note:
You must create custom environment variables beginning with "
REACT_APP_
". Any other variables will be ignored.
Example:
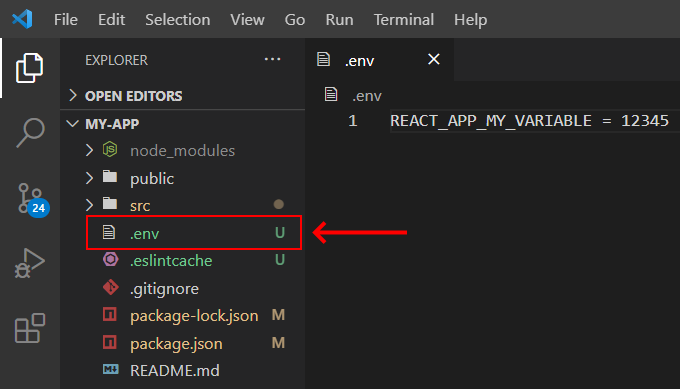
Note:
Don't store secret data inside the
.env
file because anyone can see them by inspecting your app's files.
2. Defining temporary environment variable in command line
The following examples show how to add an environment variable from the command line. This variable has higher priority over those defined in the .env
file so it will be used instead of the existing one.
Windows (cmd.exe) example:
set "REACT_APP_MY_VARIABLE=12345" && npm start
Linux, macOS (Bash) example:
REACT_APP_MY_VARIABLE=12345 npm start
Note:
If we use this approach we have to add a variable every time we start the application from cmd/bash.
3. Using environment variable
Regardless of which of the above approaches we choose, we use environment variables the same way.
These environment variables will be defined for you on process.env
, for example REACT_APP_MY_VARIABLE
will be exposed in JS as process.env.REACT_APP_MY_VARIABLE
.
Practical example:
const App = () => {
return (
<div>{process.env.REACT_APP_MY_VARIABLE}</div>
);
}