EN
React - how to style
3
points
In this article, we would like to show you how to style elements in React.
There are two of the most common ways to style in React:
- by using inline styling object with
style
property, - by using CSS style sheets with
className
property.
Note: the truth is there are more ways how to style e.g. styledComponents, etc. but in this article we present only simplest approaches.
1. Using inline styling object
In this solution we create const myComponentStyle
for our style, then we need to attach it to MyComponent
with style
property.
// ONLINE-RUNNER:browser;
// import React from 'react'
// import ReactDOM from 'react'
const myComponentStyle = {
height: '200px',
width: '200px',
background: 'goldenrod',
textAlign: 'center'
}
const MyComponent = () => {
return (
<div style={myComponentStyle}>
MyComponent
</div>
)
}
const root = document.querySelector('#root');
ReactDOM.render(<MyComponent/>, root );
Note:
We use different syntax in inline styling than in CSS style sheets (camel case convention).
2. Using CSS style sheets
In this solution, we need to attach ./MyComponent.css
file which contains my-component-style
definition that will be used by MyComponent
with className
property.
MyComponent.jsx
file:
import React from 'react'
import './MyComponent.css'
const MyComponent = () => {
return (
<div className="my-component-style">
MyComponent
</div>
)
}
MyComponent.css
file:
.my-component-style {
width: 200px;
height: 200px;
background: goldenrod;
text-align: center;
}
Browser output:
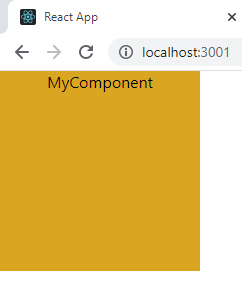