React - how to show or hide element
In this article, we would like to show you how to show or hide elements in React.
Quick solution:
import React, { useState } from 'react';
const App = () => {
const [visible, setVisible] = useState(false); // visibility state
// const showElement = () => setVisible(true);
// const hideElement = () => setVisible(false);
return (
<div>
{visible && <div>My element</div>}
</div>
);
};
There are to three ways how to show or hide elements in React:
- using conditional rendering,
- using
style
property, - using
className
property.
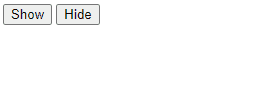
In the below examples, we use buttons that hide and show <div>My element</div>
element. We use useState()
hook to store the visibility state.
1. Conditional rendering
Conditional rendering uses {[condition-here] && (...)}
JSX construction. When state is changed the component is re-rendered. That approach causes nodes redering/omitting/removing depending on [condition-here]
value (true
/false
). The main advantage of conditional rendering is performance (DOM doesn't contain unused nodes).
1.1. One button solution
When onClick
button event occurs we are able to set new visible
state with setVisible(!visible)
- clicks hide and show the element.
Practical example:
// ONLINE-RUNNER:browser;
// Note: Uncomment import lines during working with JSX Compiler.
// import React from 'react';
const App = () => {
const [visible, setVisible] = React.useState(false);
return (
<div>
<button onClick={() => setVisible(!visible)}>{visible ? 'Hide' : 'Show'}</button>
{visible && <div>My element</div>}
</div>
);
};
const root = document.querySelector('#root');
ReactDOM.render(<App />, root );
1.2. Two buttons solution
One buton is used to display element and second one to hide the same element.
Practical example:
// ONLINE-RUNNER:browser;
// Note: Uncomment import lines during working with JSX Compiler.
// import React from 'react';
const App = () => {
const [visible, setVisible] = React.useState(false);
return (
<div>
<button onClick={() => setVisible(true)}>Show</button>
<button onClick={() => setVisible(false)}>Hide</button>
{visible && <div>My element</div>}
</div>
);
};
const root = document.querySelector('#root');
ReactDOM.render(<App />, root );
2. style
property
style
property uses <div style={{display: [condition-here] ? 'block' : 'none'>...</div>
JSX construction. In this case the element is not removed - it is just invisible by styles.
Practical example:
// ONLINE-RUNNER:browser;
// Note: Uncomment import lines during working with JSX Compiler.
// import React from 'react';
const App = () => {
const [visible, setVisible] = React.useState(false);
return (
<div>
<button onClick={() => setVisible(!visible)}>{visible ? 'Hide' : 'Show'}</button>
<div style={{display: visible ? 'block' : 'none'}}>My element</div>
</div>
);
};
const root = document.querySelector('#root');
ReactDOM.render(<App />, root );
3. className
property
className
property uses <div className={[condition-here] ? 'class-1' : 'class-2'>...</div>
JSX construction. In this case the element is not removed - it is just invisible by styles.
Practical example:
// ONLINE-RUNNER:browser;
// Note: Uncomment import lines during working with JSX Compiler.
// import React from 'react';
const App = () => {
const [visible, setVisible] = React.useState(false);
return (
<div>
<style>{`
.element-visible { display: block }
.element-hidden { display: none }
`}</style>
<button onClick={() => setVisible(!visible)}>{visible ? 'Hide' : 'Show'}</button>
<div className={visible ? 'element-visible' : 'element-hidden'}>My element</div>
</div>
);
};
const root = document.querySelector('#root');
ReactDOM.render(<App />, root );