PL
JavaScript - Math.log2() - przykład metody z dokumentacją
6 points
Math.log2()
ta metoda zwraca logarytm o podstawie 2
liczby.
xxxxxxxxxx
1
// Logarithm with base 2:
2
// x y
3
console.log( Math.log2( 1 ) ); // 0
4
console.log( Math.log2( 2 ) ); // 1
5
console.log( Math.log2( 4 ) ); // 2
6
console.log( Math.log2( 8 ) ); // 3
7
console.log( Math.log2( 1024 ) ); // 10
8
9
console.log( Math.log2( -1 ) ); // NaN
10
console.log( Math.log2( 0 ) ); // -Infinity
11
console.log( Math.log2( +Infinity ) ); // +Infinity
12
Metodę Math.log2()
przedstawia poniższy wykres:
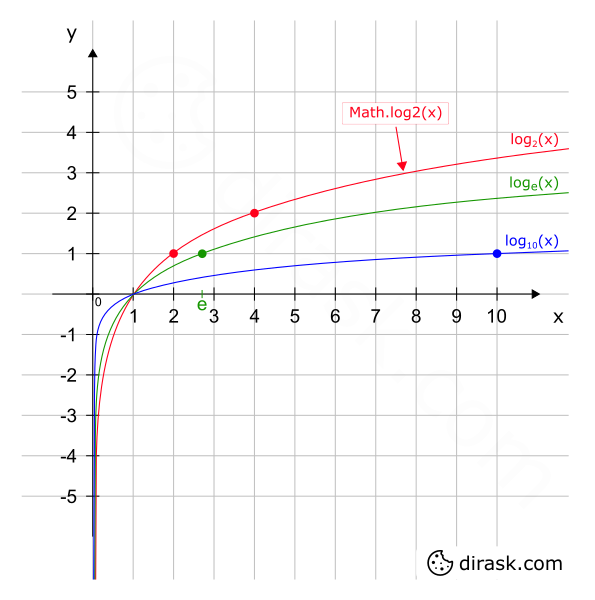
Składnia | Math.log2(x) |
Parametry | x - liczba całkowita lub zmiennoprzecinkowa w zakresie 0 do +Infinity (wartość prymitywna). |
Wynik |
Jeżeli Jeżeli Jeżeli |
Opis |
|
xxxxxxxxxx
1
if (Math.log2 == null) {
2
Math.log2 = function(x) {
3
/*
4
1. Using logarithm definition:
5
b^y = x <=> log_b(x) = y
6
Where: b - base
7
8
2. We can create system of equations:
9
y = log_b(x)
10
x = b^y
11
12
3. Which can be expressed as:
13
x = b^log_b(x)
14
15
4. We can transform equation:
16
log_2(x) = log_2(e^log_e(x)) = log_2(e) * log_e(x) = log_e(x) * log_2(e)
17
18
5. It can be written in JavaScript:
19
log_2(e) <=> Math.LOG2E
20
log_e(x) * log_2(e) <=> Math.log(x) * Math.LOG2E
21
*/
22
return Math.log(x) * Math.LOG2E;
23
};
24
}
25
26
// Usage example:
27
// x y
28
console.log( Math.log2( 1 ) ); // 0
29
console.log( Math.log2( 2 ) ); // 1
30
console.log( Math.log2( 4 ) ); // ~2
31
console.log( Math.log2( 8 ) ); // 3
32
console.log( Math.log2( 1024 ) ); // 10
33
34
console.log( Math.log2( -1 ) ); // NaN
35
console.log( Math.log2( 0 ) ); // -Infinity
36
console.log( Math.log2( +Infinity ) ); // +Infinity
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style> #canvas { border: 1px solid black; } </style>
5
</head>
6
<body>
7
<canvas id="canvas" width="400" height="400"></canvas>
8
<script>
9
10
var canvas = document.querySelector('#canvas');
11
var context = canvas.getContext('2d');
12
13
// logarithm chart range
14
var x1 = 0;
15
var x2 = 10;
16
var y1 = -5;
17
var y2 = 4;
18
19
var dx = 0.005;
20
21
var xRange = x2 - x1;
22
var yRange = y2 - y1;
23
24
function calculateLogarithm(base, x) {
25
var a = Math.log(x);
26
var b = Math.log(base);
27
28
return a / b;
29
}
30
31
function calculatePoint(base, x) {
32
var y = calculateLogarithm(base, x);
33
34
// wykres zostanie odwrócony w poziomie z powodu odwróconych pikseli obszaru roboczego
35
36
var nx = (x - x1) / xRange; // normalized x
37
var ny = 1.0 - (y - y1) / yRange; // normalized y
38
39
var point = {
40
x: nx * canvas.width,
41
y: ny * canvas.height
42
};
43
44
return point;
45
}
46
47
function drawChart(base, color, thickness) {
48
var point = calculatePoint(base, x1);
49
50
context.beginPath();
51
context.lineWidth = thickness;
52
context.strokeStyle = color;
53
context.moveTo(point.x, point.y);
54
55
for (var x = x1 + dx; x < x2; x += dx) {
56
point = calculatePoint(base, x);
57
context.lineTo(point.x, point.y);
58
}
59
60
point = calculatePoint(base, x2);
61
context.lineTo(point.x, point.y);
62
context.stroke();
63
}
64
65
console.log('log_2(x) <- red');
66
console.log('log_e(x) <- green');
67
console.log('log_10(x) <- blue');
68
69
// base color thickness
70
drawChart( 2, '#ff001b', 2 ); // red
71
drawChart( Math.E, '#159600', 0.8 ); // green
72
drawChart( 10, '#0000ff', 0.8 ); // blue
73
74
console.log('x range: <' + x1 + '; ' + x2 + '>');
75
console.log('y range: <' + y1 + '; ' + y2 + '>');
76
77
</script>
78
</body>
79
</html>