EN
CSS - justify content in flexbox (flex-direction: column)
4 points
In this article, we would like to show you how to justify content inside flex container in CSS, when flex-direction
is set to column
.
Note: to see the same effect in row flex direction check this article.
Working with flexbox, we can set justify-content
property to the following values:
flex-start
flex-end
center
space-between
space-around
space-evenly
Following property organize items in the following way when flex-direction
style property is set to column
value.
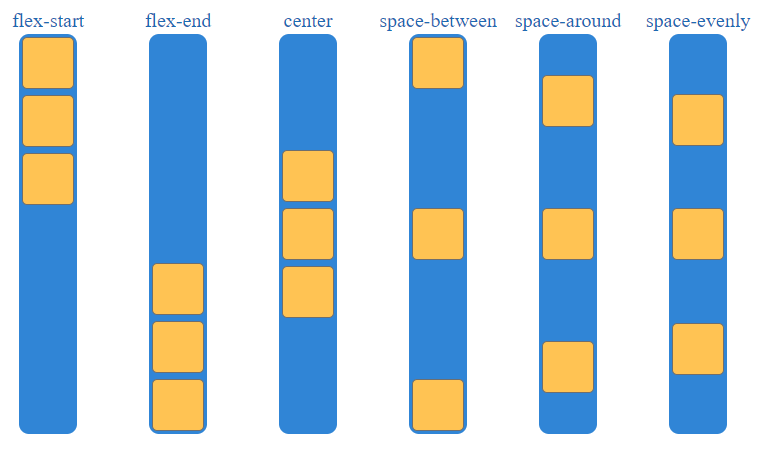
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
6
.container {
7
margin: 2px auto 0 auto;
8
border-radius: 10px;
9
background: #3085d6;
10
width: 58px;
11
height: 400px;
12
display: flex; /* <-------------- required */
13
flex-direction: column; /* <-------------- required */
14
justify-content: flex-start; /* <-------------- required */
15
}
16
17
.item {
18
margin: 3px;
19
border: 1px solid #6e6e6e;
20
border-radius: 5px;
21
background: #ffc353;
22
height: 50px;
23
width: 50px;
24
}
25
26
</style>
27
</head>
28
<body>
29
<div class="container">
30
<div class="item"></div>
31
<div class="item"></div>
32
<div class="item"></div>
33
</div>
34
</body>
35
</html>
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
6
.container {
7
margin: 2px auto 0 auto;
8
border-radius: 10px;
9
background: #3085d6;
10
width: 58px;
11
height: 400px;
12
display: flex; /* <-------------- required */
13
flex-direction: column; /* <-------------- required */
14
justify-content: flex-end; /* <-------------- required */
15
}
16
17
.item {
18
margin: 3px;
19
border: 1px solid #6e6e6e;
20
border-radius: 5px;
21
background: #ffc353;
22
height: 50px;
23
width: 50px;
24
}
25
26
</style>
27
</head>
28
<body>
29
<div class="container">
30
<div class="item"></div>
31
<div class="item"></div>
32
<div class="item"></div>
33
</div>
34
</body>
35
</html>
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
6
.container {
7
margin: 2px auto 0 auto;
8
border-radius: 10px;
9
background: #3085d6;
10
width: 58px;
11
height: 400px;
12
display: flex; /* <-------------- required */
13
flex-direction: column; /* <-------------- required */
14
justify-content: center; /* <-------------- required */
15
}
16
17
.item {
18
margin: 3px;
19
border: 1px solid #6e6e6e;
20
border-radius: 5px;
21
background: #ffc353;
22
height: 50px;
23
width: 50px;
24
}
25
26
</style>
27
</head>
28
<body>
29
<div class="container">
30
<div class="item"></div>
31
<div class="item"></div>
32
<div class="item"></div>
33
</div>
34
</body>
35
</html>
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
6
.container {
7
margin: 2px auto 0 auto;
8
border-radius: 10px;
9
background: #3085d6;
10
width: 58px;
11
height: 400px;
12
display: flex; /* <-------------- required */
13
flex-direction: column; /* <-------------- required */
14
justify-content: space-between; /* <-------------- required */
15
}
16
17
.item {
18
margin: 3px;
19
border: 1px solid #6e6e6e;
20
border-radius: 5px;
21
background: #ffc353;
22
height: 50px;
23
width: 50px;
24
}
25
26
</style>
27
</head>
28
<body>
29
<div class="container">
30
<div class="item"></div>
31
<div class="item"></div>
32
<div class="item"></div>
33
</div>
34
</body>
35
</html>
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
6
.container {
7
margin: 2px auto 0 auto;
8
border-radius: 10px;
9
background: #3085d6;
10
width: 58px;
11
height: 400px;
12
display: flex; /* <-------------- required */
13
flex-direction: column; /* <-------------- required */
14
justify-content: space-around; /* <-------------- required */
15
}
16
17
.item {
18
margin: 3px;
19
border: 1px solid #6e6e6e;
20
border-radius: 5px;
21
background: #ffc353;
22
height: 50px;
23
width: 50px;
24
}
25
26
</style>
27
</head>
28
<body>
29
<div class="container">
30
<div class="item"></div>
31
<div class="item"></div>
32
<div class="item"></div>
33
</div>
34
</body>
35
</html>
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
6
.container {
7
margin: 2px auto 0 auto;
8
border-radius: 10px;
9
background: #3085d6;
10
width: 58px;
11
height: 400px;
12
display: flex; /* <-------------- required */
13
flex-direction: column; /* <-------------- required */
14
justify-content: space-evenly; /* <-------------- required */
15
}
16
17
.item {
18
margin: 3px;
19
border: 1px solid #6e6e6e;
20
border-radius: 5px;
21
background: #ffc353;
22
height: 50px;
23
width: 50px;
24
}
25
26
</style>
27
</head>
28
<body>
29
<div class="container">
30
<div class="item"></div>
31
<div class="item"></div>
32
<div class="item"></div>
33
</div>
34
</body>
35
</html>