EN
Node.js - PostgreSQL Inner Join
0 points
In this article, we would like to show you how to use SQL INNER JOIN in Node.js.
Note: at the end of this article you can find database preparation SQL queries.
xxxxxxxxxx
1
const { Client } = require('pg');
2
3
const client = new Client({
4
host: '127.0.0.1',
5
user: 'postgres',
6
database: 'database_name',
7
password: 'password',
8
port: 5432,
9
});
10
11
const fetchUserDepartments = async () => {
12
const query = `SELECT *
13
FROM "users"
14
JOIN "departments" ON "departments"."id" = "users"."department_id"`;
15
try {
16
await client.connect(); // gets connection
17
const { rows } = await client.query(query); // sends queries
18
console.table(rows);
19
} catch (error) {
20
console.error(error.stack);
21
} finally {
22
await client.end(); // closes connection
23
}
24
};
25
26
fetchUserDepartments();
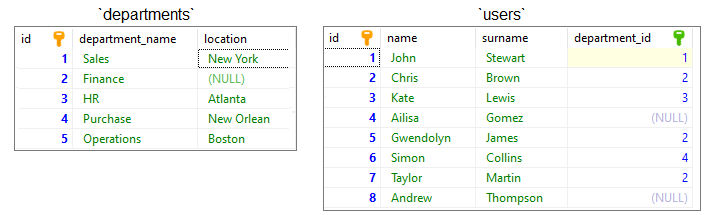
Output:
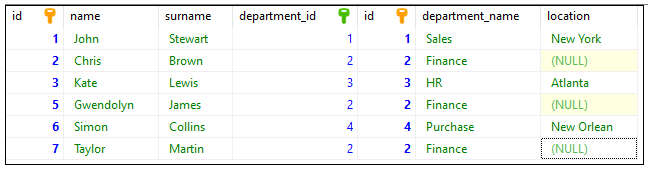
create_tables.sql
file:
xxxxxxxxxx
1
CREATE TABLE "departments" (
2
"id" SERIAL,
3
"department_name" VARCHAR(50) NOT NULL,
4
"location" VARCHAR(50) NULL,
5
PRIMARY KEY ("id")
6
);
7
8
CREATE TABLE "users" (
9
"id" SERIAL,
10
"name" VARCHAR(50) NOT NULL,
11
"surname" VARCHAR(50) NOT NULL,
12
"department_id" INTEGER,
13
PRIMARY KEY ("id"),
14
FOREIGN KEY ("department_id") REFERENCES "departments" ("id")
15
);
insert_data.sql
file:
xxxxxxxxxx
1
INSERT INTO "departments"
2
("id", "department_name", "location")
3
VALUES
4
(1, 'Sales', 'New York'),
5
(2, 'Finance', NULL),
6
(3, 'HR', 'Atlanta'),
7
(4, 'Purchase', 'New Orlean'),
8
(5, 'Operations', 'Boston');
9
10
INSERT INTO "users"
11
( "name", "surname", "department_id")
12
VALUES
13
('John', 'Stewart', 1),
14
('Chris', 'Brown', 2),
15
('Kate', 'Lewis', 3),
16
('Ailisa', 'Gomez', NULL),
17
('Gwendolyn', 'James', 2),
18
('Simon', 'Collins', 4),
19
('Taylor', 'Martin', 2),
20
('Andrew', 'Thompson', NULL);