EN
Node.js - PostgreSQL CREATE TABLE AS
0
points
In this article, we would like to show you how to create a new table from a query using CREATE TABLE AS statement in the Postgres database from Node.js level.
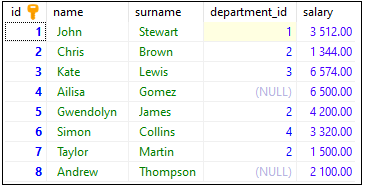
Note: at the end of this article you can find database preparation SQL queries.
const { Client } = require('pg');
const client = new Client({
host: '127.0.0.1',
user: 'my_username',
database: 'my_database',
password: 'my_password',
port: 5432,
});
const newTableFromQuery = async () => {
const query = `
CREATE TABLE "users_without_email" AS
SELECT *
FROM "users"
WHERE "email" IS NULL;
`;
await client.connect(); // creates connection
try {
await client.query(query); // sends query
} finally {
await client.end(); // closes connection
}
};
newTableFromQuery()
.then(() => console.table('New table created!'))
.catch(error => console.error(error.stack));
Result:
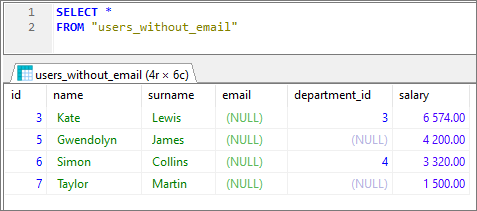
Database preparation
create_tables.sql
file:
CREATE TABLE "users" (
"id" SERIAL,
"name" VARCHAR(50) NOT NULL,
"surname" VARCHAR(50) NOT NULL,
"email" VARCHAR(100),
"department_id" INTEGER,
"salary" DECIMAL(15,2) NOT NULL,
PRIMARY KEY ("iusers_without_emaild")
);
insert_data.sql
file:
INSERT INTO "users"
( "name", "surname", "email", "department_id", "salary")
VALUES
('John', 'Stewart', 'john@email.com', 1, '3512.00'),
('Chris', 'Brown', 'chris@email.com', 2, '1344.00'),
('Kate', 'Lewis', NULL, 3, '6574.00'),
('Ailisa', 'Gomez', 'ailisa@email.com', 2, '6500.00'),
('Gwendolyn', 'James', NULL, NULL, '4200.00'),
('Simon', 'Collins', NULL, 4, '3320.00'),
('Taylor', 'Martin', NULL, NULL, '1500.00'),
('Andrew', 'Thompson', 'andrew@email.com', NULL, '2100.00');
Native SQL query (used in the above example):
CREATE TABLE "users_without_email1" AS
SELECT *
FROM "users"
WHERE "email" IS NULL;