EN
Node.js - PostgreSQL - rows pagination
3
points
In this article, we would like to show you how to paginate data rows in the PostgreSQL using Node.js.
Note:
Pagination consists in dividing data into pages and sending only those rows that the client currently needs - we do not have to send all data at once.
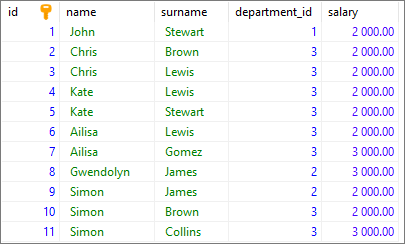
Note: at the end of this article you can find database preparation SQL queries.
const { Client } = require('pg');
const client = new Client({
host: '127.0.0.1',
user: 'my_username',
database: 'my_database',
password: 'my_password',
port: 5432,
});
const fetchRows = async (pageNumber, pageSize) => {
const query = `
SELECT *
FROM "users"
ORDER BY "users"."id"
LIMIT $2
OFFSET (($1 - 1) * $2);
`;
await client.connect(); // creates connection
try {
const { rows } = await client.query(query, [pageNumber, pageSize]); // sends query
return rows;
} finally {
await client.end(); // closes connection
}
};
fetchRows(1, 3) // fetch 3 rows from page 1
.then(result => console.table(result))
.catch(error => console.error(error.stack));
Result:
┌─────────┬────┬─────────┬───────────┬───────────────┬───────────┐
│ (index) │ id │ name │ surname │ department_id │ salary │
├─────────┼────┼─────────┼───────────┼───────────────┼───────────┤
│ 0 │ 1 │ 'John' │ 'Stewart' │ 1 │ '2000.00' │
│ 1 │ 2 │ 'Chris' │ 'Brown' │ 3 │ '2000.00' │
│ 2 │ 3 │ 'Chris' │ 'Lewis' │ 3 │ '2000.00' │
└─────────┴────┴─────────┴───────────┴───────────────┴───────────┘
Result for fetchRows(2, 4)
:
┌─────────┬────┬─────────────┬───────────┬───────────────┬───────────┐
│ (index) │ id │ name │ surname │ department_id │ salary │
├─────────┼────┼─────────────┼───────────┼───────────────┼───────────┤
│ 0 │ 5 │ 'Kate' │ 'Stewart' │ 3 │ '2000.00' │
│ 1 │ 6 │ 'Ailisa' │ 'Lewis' │ 3 │ '2000.00' │
│ 2 │ 7 │ 'Ailisa' │ 'Gomez' │ 3 │ '3000.00' │
│ 3 │ 8 │ 'Gwendolyn' │ 'James' │ 2 │ '3000.00' │
└─────────┴────┴─────────────┴───────────┴───────────────┴───────────┘
Database preparation
create_tables.sql
file:
CREATE TABLE "users" (
"id" SERIAL,
"name" VARCHAR(50) NOT NULL,
"surname" VARCHAR(50) NOT NULL,
"department_id" INTEGER,
"salary" DECIMAL(15,2) NOT NULL,
PRIMARY KEY ("id")
);
insert_data.sql
file:
INSERT INTO "users"
( "name", "surname", "department_id", "salary")
VALUES
('John', 'Stewart', 1, '2000.00'),
('Chris', 'Brown', 3, '2000.00'),
('Chris', 'Lewis', 3, '2000.00'),
('Kate', 'Lewis', 3, '2000.00'),
('Kate', 'Stewart', 3, '2000.00'),
('Ailisa', 'Lewis', 3, '2000.00'),
('Ailisa', 'Gomez', 3, '3000.00'),
('Gwendolyn', 'James', 2, '3000.00'),
('Simon', 'James', 2, '2000.00'),
('Simon', 'Brown', 3, '2000.00'),
('Simon', 'Collins', 3, '3000.00');
Native SQL query (used in the above example):
SELECT *
FROM "users"
ORDER BY "users"."id"
LIMIT 4
OFFSET 1 * 4;