EN
CSS - how to crop / clip and resize image
5
points
Using CSS it is possible to crop and resize image in few ways.
1. clip: rect()
property example
Most important things about this approach are:
- it uses
clip: rect()
style property to hide (likevisibility: hidden
for part of element) unwanted part of image, - after clipping it is necessary to correct position of clipped element,
clip
property works withposition: absolute
andposition: fixed
properties, so it is necessary to use too,- image resize effect is achived with
width: 100px
andheigh: 100px
properties, clip: rect()
property workd for other html elements too.
Main concept of how clip
property works has been described below:
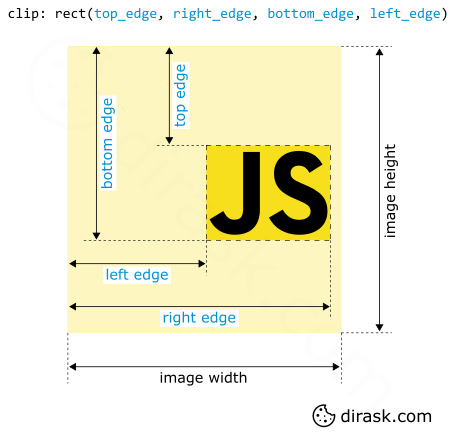
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<style>
body {
position: relative;
height: 70px;
}
.cropped-image {
position: absolute; /* required property for clip */
left: -24px; /* -left_edge */
top: -43px; /* -top_edge */
width: 100px; /* image_scaled_width */
height: 100px; /* image_scaled_height */
/* edges: top_edge, right_edge, bottom_edge, left_edge */
clip: rect(43px, 95px, 95px, 24px);
}
</style>
</head>
<body>
<img class="cropped-image"
src="//dirask.com/static/bucket/1574890428058-BZOQxN2D3p--image.png" />
</body>
</html>
2. Element with rescaled content examples
This examples show how to achive same clipping effect to above example, but using overflow: hidden
style property.
2.1. With shifted margin and background resising
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<style>
.crop-container {
border: 1px solid black;
width: 71px; /* 95px - 24px (right_edge - left_edge) */
height: 52px; /* 95px - 43px (bottom_edge - top_edge ) */
overflow: hidden;
}
.cropped-image {
/* -left_edge -top_edge */
margin: -43px 0 0 -24px;
width: 100px; /* image_scaled_width */
height: 100px; /* image_scaled_height */
background: url('//dirask.com/static/bucket/1574890428058-BZOQxN2D3p--image.png')
/* image_scaled_width image_scaled_height */
no-repeat 0 / 100px 100px;
}
</style>
</head>
<body>
<div class="crop-container">
<div class="cropped-image" />
</div>
</body>
</html>
2.2. With shifter margin and img element resizing
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<style>
.crop-container {
border: 1px solid black;
width: 71px; /* 95px - 24px (right_edge - left_edge) */
height: 52px; /* 95px - 43px (bottom_edge - top_edge ) */
overflow: hidden;
}
.cropped-image {
/* -left_edge -top_edge */
margin: -43px 0 0 -24px;
width: 100px; /* image_scaled_width */
height: 100px; /* image_scaled_height */
}
</style>
</head>
<body>
<div class="crop-container">
<img class="cropped-image"
src="//dirask.com/static/bucket/1574890428058-BZOQxN2D3p--image.png" />
</div>
</body>
</html>
2.3. With shifted position and background resising
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<style>
.crop-container {
position: relative;
border: 1px solid black;
width: 71px; /* 95px - 24px (right_edge - left_edge) */
height: 52px; /* 95px - 43px (bottom_edge - top_edge ) */
overflow: hidden;
}
.cropped-image {
position: absolute;
left: -24px; /* -left_edge */
top: -43px; /* -top_edge */
width: 100px; /* image_scaled_width */
height: 100px; /* image_scaled_height */
background: url('//dirask.com/static/bucket/1574890428058-BZOQxN2D3p--image.png')
/* image_scaled_width image_scaled_height */
no-repeat 0 / 100px 100px;
}
</style>
</head>
<body>
<div class="crop-container">
<div class="cropped-image" />
</div>
</body>
</html>
2.3. With shifted position and img element resizing
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<style>
.crop-container {
position: relative;
border: 1px solid black;
width: 71px; /* 95px - 24px (right_edge - left_edge) */
height: 52px; /* 95px - 43px (bottom_edge - top_edge ) */
overflow: hidden;
}
.cropped-image {
position: absolute;
left: -24px; /* -left_edge */
top: -43px; /* -top_edge */
width: 100px; /* image_scaled_width */
height: 100px; /* image_scaled_height */
}
</style>
</head>
<body>
<div class="crop-container">
<img class="cropped-image"
src="//dirask.com/static/bucket/1574890428058-BZOQxN2D3p--image.png" />
</div>
</body>
</html>
Used resources:
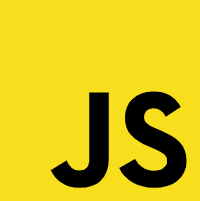
See also
Merged questions