EN
Node.js - PostgreSQL ALTER TABLE - Add column
0
points
In this article, we would like to show you how to add a column to the existing table by ALTER TABLE in the Postgres database using Node.js.
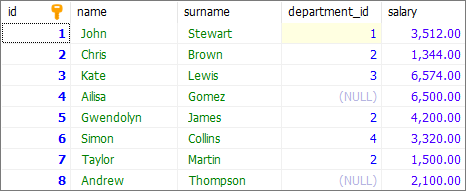
Note: at the end of this article you can find database preparation SQL queries.
const { Client } = require('pg');
const client = new Client({
host: '127.0.0.1',
user: 'postgres',
database: 'database_name',
password: 'password',
port: 5432,
});
const addColumn = async () => {
const query = `ALTER TABLE "users"
ADD "email" VARCHAR(255);`;
try {
await client.connect(); // gets connection
await client.query(query); // sends query
} catch (error) {
console.error(error.stack);
} finally {
await client.end(); // closes connection
}
};
addColumn();
Result:
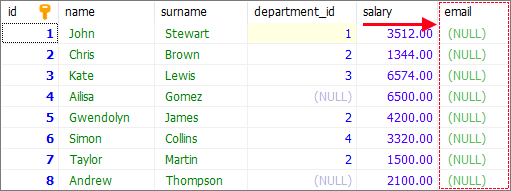
Database preparation
create_tables.sql
file:
CREATE TABLE "users" (
"id" SERIAL,
"name" VARCHAR(50) NOT NULL,
"surname" VARCHAR(50) NOT NULL,
"department_id" INTEGER,
"salary" DECIMAL(15,2) NOT NULL,
PRIMARY KEY ("id")
);
insert_data.sql
file:
INSERT INTO "users"
( "name", "surname", "department_id", "salary")
VALUES
('John', 'Stewart', 1, '3512.00'),
('Chris', 'Brown', 2, '1344.00'),
('Kate', 'Lewis', 3, '6574.00'),
('Ailisa', 'Gomez', NULL, '6500.00'),
('Gwendolyn', 'James', 2, '4200.00'),
('Simon', 'Cousersllins', 4, '3320.00'),
('Taylor', 'Martin', 2, '1500.00'),
('Andrew', 'Thompson', NULL, '2100.00');
Native SQL query (used in the above example):
ALTER TABLE `users`
ADD `email` VARCHAR(255);