EN
Node.js - PostgreSQL LIKE operator
0
points
In this article, we would like to show you how to use Postgres LIKE operator in Node.js.
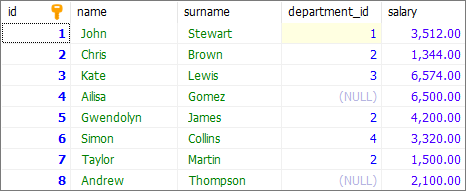
Note: at the end of this article you can find database preparation SQL queries.
const { Client } = require('pg');
const client = new Client({
host: '127.0.0.1',
user: 'my_username',
database: 'my_database',
password: 'my_password',
port: 5432,
});
const findRows = async (pattern) => {
const query = `
SELECT * FROM "users"
WHERE "name" LIKE $1;
`;
await client.connect(); // creates connection
try {
const { rows } = await client.query(query, [pattern]); // sends query
return rows;
} finally {
await client.end(); // closes connection
}
};
findRows('A%') // Ailisa, Andrew
.then(result => console.table(result))
.catch(error => console.error(error.stack));
Result:
┌─────────┬────┬──────────┬────────────┬───────────────┬───────────┐
│ (index) │ id │ name │ surname │ department_id │ salary │
├─────────┼────┼──────────┼────────────┼───────────────┼───────────┤
│ 0 │ 4 │ 'Ailisa' │ 'Gomez' │ null │ '6500.00' │
│ 1 │ 8 │ 'Andrew' │ 'Thompson' │ null │ '2100.00' │
└─────────┴────┴──────────┴────────────┴───────────────┴───────────┘
Database preparation
create_tables.sql
file:
CREATE TABLE "users" (
"id" SERIAL,
"name" VARCHAR(50) NOT NULL,
"surname" VARCHAR(50) NOT NULL,
"department_id" INTEGER,
"salary" DECIMAL(15,2) NOT NULL,
PRIMARY KEY ("id")
);
insert_data.sql
file:
INSERT INTO "users"
( "name", "surname", "department_id", "salary")
VALUES
('John', 'Stewart', 1, '3512.00'),
('Chris', 'Brown', 2, '1344.00'),
('Kate', 'Lewis', 3, '6574.00'),
('Ailisa', 'Gomez', NULL, '6500.00'),
('Gwendolyn', 'James', 2, '4200.00'),
('Simon', 'Cousersllins', 4, '3320.00'),
('Taylor', 'Martin', 2, '1500.00'),
('Andrew', 'Thompson', NULL, '2100.00');
Native SQL query (used in the above example):
SELECT * FROM "users"
WHERE "name" LIKE 'A%';