EN
Java - get file size
3
points
In this article, we would like to show you how to get file size in Java.
Below we present 3 methods of getting the file size:
File.length()
Files.size()
FileChannel.size()
1. Using File.length()
method.
import java.io.File;
public class Example {
public static void main(String[] args) {
File file = new File("C:\\projects\\file.txt");
long fileSize = file.length();
System.out.println("File size in bytes: " + fileSize + " B");
System.out.println("File size in kilobytes: " + fileSize / 1024 + " KB");
System.out.println("File size in megabytes: " + fileSize / (1024 * 1024) + " MB");
}
}
Output:
File size in bytes: 113804 B
File size in kilobytes: 111 KB
File size in megabytes: 0 MB
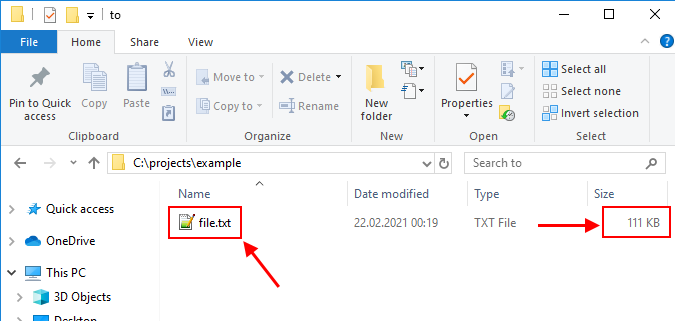
2. Using Files.size()
method.
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class Example {
public static void main(String[] args) throws IOException {
Path filePath = Paths.get("C:\\projects\\file.txt");
long fileSize = Files.size(filePath);
System.out.println("File size in bytes: " + fileSize + " B");
System.out.println("File size in kilobytes: " + fileSize / 1024 + " KB");
System.out.println("File size in megabytes: " + fileSize / (1024 * 1024) + " MB");
}
}
Output:
File size in bytes: 113804 B
File size in kilobytes: 111 KB
File size in megabytes: 0 MB
3. Using FileChannel.size()
method.
import java.io.IOException;
import java.nio.channels.FileChannel;
import java.nio.file.Path;
import java.nio.file.Paths;
public class Example {
public static void main(String[] args) throws IOException {
Path filePath = Paths.get("C:\\projects\\file.txt");
FileChannel fileChannel = FileChannel.open(filePath);
long fileSize = fileChannel.size();
System.out.println("File size in bytes: " + fileSize + " B");
System.out.println("File size in kilobytes: " + fileSize / 1024 + " KB");
System.out.println("File size in megabytes: " + fileSize / (1024 * 1024) + " MB");
}
}
Output:
File size in bytes: 113804 B
File size in kilobytes: 111 KB
File size in megabytes: 0 MB