EN
Java - get file size
3 points
In this article, we would like to show you how to get file size in Java.
Below we present 3 methods of getting the file size:
File.length()
Files.size()
FileChannel.size()
xxxxxxxxxx
1
import java.io.File;
2
3
public class Example {
4
5
public static void main(String[] args) {
6
File file = new File("C:\\projects\\file.txt");
7
long fileSize = file.length();
8
9
System.out.println("File size in bytes: " + fileSize + " B");
10
System.out.println("File size in kilobytes: " + fileSize / 1024 + " KB");
11
System.out.println("File size in megabytes: " + fileSize / (1024 * 1024) + " MB");
12
}
13
}
Output:
xxxxxxxxxx
1
File size in bytes: 113804 B
2
File size in kilobytes: 111 KB
3
File size in megabytes: 0 MB
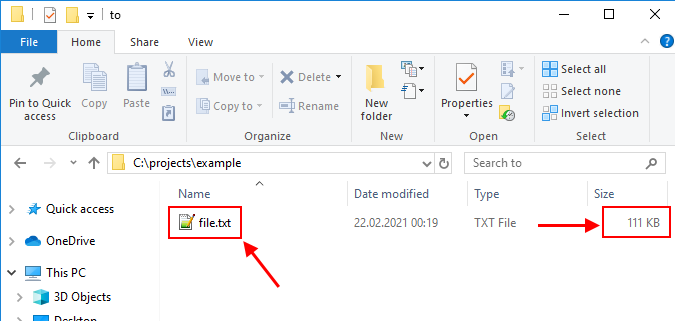
xxxxxxxxxx
1
import java.io.IOException;
2
import java.nio.file.Files;
3
import java.nio.file.Path;
4
import java.nio.file.Paths;
5
6
public class Example {
7
8
public static void main(String[] args) throws IOException {
9
Path filePath = Paths.get("C:\\projects\\file.txt");
10
long fileSize = Files.size(filePath);
11
12
System.out.println("File size in bytes: " + fileSize + " B");
13
System.out.println("File size in kilobytes: " + fileSize / 1024 + " KB");
14
System.out.println("File size in megabytes: " + fileSize / (1024 * 1024) + " MB");
15
}
16
}
Output:
xxxxxxxxxx
1
File size in bytes: 113804 B
2
File size in kilobytes: 111 KB
3
File size in megabytes: 0 MB
xxxxxxxxxx
1
import java.io.IOException;
2
import java.nio.channels.FileChannel;
3
import java.nio.file.Path;
4
import java.nio.file.Paths;
5
6
public class Example {
7
8
public static void main(String[] args) throws IOException {
9
Path filePath = Paths.get("C:\\projects\\file.txt");
10
FileChannel fileChannel = FileChannel.open(filePath);
11
long fileSize = fileChannel.size();
12
13
System.out.println("File size in bytes: " + fileSize + " B");
14
System.out.println("File size in kilobytes: " + fileSize / 1024 + " KB");
15
System.out.println("File size in megabytes: " + fileSize / (1024 * 1024) + " MB");
16
}
17
}
Output:
xxxxxxxxxx
1
File size in bytes: 113804 B
2
File size in kilobytes: 111 KB
3
File size in megabytes: 0 MB