Java - append text to existing file
In this article, we would like to show you how to append text to an existing file in Java.
Below examples present how to append text into the file using the following solutions:
Files.write
(append single line - Java 7, multiple lines - Java 8),Files.writeString
(Java 11),FileWriter
,FileOutputStream
.
1. Files.write
In the below examples, we use Files.write
to append some text at the end of the existing example.txt
file.
1.1 Append single line
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardOpenOption;
public class Example {
public static void main(String[] args) throws IOException {
Path path = Paths.get("C:\\path\\example.txt");
String string = "Dirask is awesome!\n";
Files.write(path, string.getBytes(StandardCharsets.UTF_8),
StandardOpenOption.CREATE,
StandardOpenOption.APPEND);
}
}
The result after three executions:
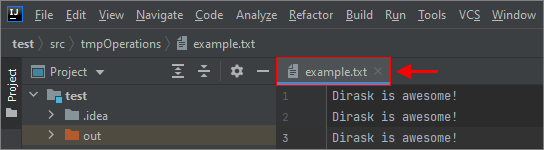
1.2 Append multiple lines
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardOpenOption;
import java.util.ArrayList;
import java.util.List;
public class Example {
public static void main(String[] args) throws IOException {
Path path = Paths.get("C:\\path\\example.txt");
List<String> text = new ArrayList<>();
text.add("line 1");
text.add("line 2");
text.add("line 3");
Files.write(path, text,
StandardOpenOption.CREATE,
StandardOpenOption.APPEND);
}
}
The result after one execution:
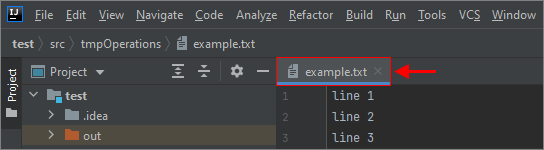
2. Files.writeString
In the below examples, we use Files.writeString
to append some text at the end of the existing example.txt
file.
Note:
In Java 7 with
Files.write()
method, we need to convert aString
into abyte[]
and then write or append it to a file.With Java 11
File.writeString()
method we can directly write or append the string into the file.
Practical example:
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardOpenOption;
public class Example {
public static void main(String[] args) throws IOException {
Path path = Paths.get("C:\\path\\example.txt");
String text = "Dirask is awesome!";
Files.writeString(path, text,
StandardOpenOption.CREATE,
StandardOpenOption.APPEND);
}
}
Result:
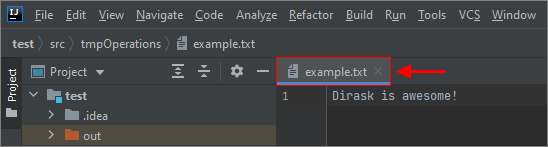
3. FileWriter
In the below examples, we use FileWriter
to append some text at the end of the existing example.txt
file.
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
public class Example {
public static void main(String[] args) throws IOException {
File file = new File("C:\\path\\example.txt");
List<String> text = new ArrayList<>();
text.add("line 1");
text.add("line 2");
text.add("line 3");
try (FileWriter fw = new FileWriter(file, true);
BufferedWriter bw = new BufferedWriter(fw)) {
for (String s : text) {
bw.write(s);
bw.newLine();
}
}
}
}
The result after one execution:
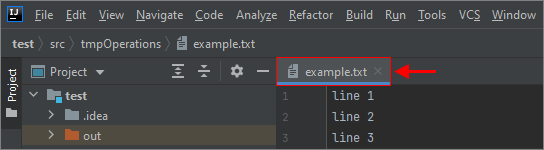
4. FileOutputStream
In the below examples, we use FileWriter
to append some text at the end of the existing example.txt
file.
import java.io.*;
import java.nio.charset.StandardCharsets;
public class Example {
public static void main(String[] args) throws IOException {
File file = new File("C:\\path\\example.txt");
String text = "Dirask is awesome!\n";
try (FileOutputStream fos = new FileOutputStream(file, true)) {
fos.write(text.getBytes(StandardCharsets.UTF_8));
}
}
}
Result:
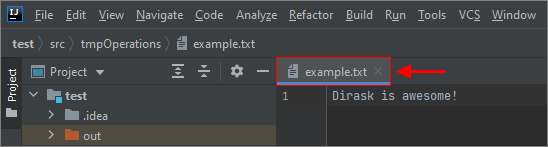
Note:
Append mode of
FileOutputStream
works the same as
FileWriter
.