EN
Java - list all files from directory
0
points
In this article, we would like to show you how to list all file names from the directory in Java.
Quick solution:
File[] files = new File(path).listFiles(File::isFile); // get array of files
Practical example
In this example, we use listFiles()
method and check if the file is a file (not a directory) to list only files from the directory specified by path
.
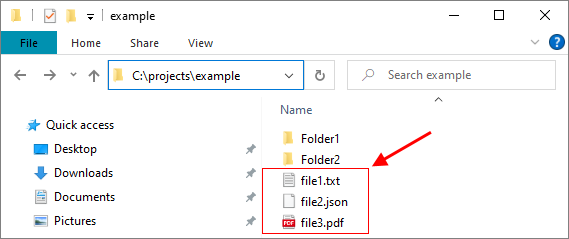
Code example:
import java.io.File;
import java.util.Objects;
public class Example {
public static void main(String[] args) {
String path = "C:\\projects\\example";
File[] files = new File(path).listFiles(File::isFile); // array of files
File[] list = Objects.requireNonNull(files); // creates list if files!= null
for (File item : list) {
System.out.println(item.getName());
}
}
}
Result:
file1.txt
file2.json
file3.pdf
Note:
To get full file paths instead of file names remove
getName()
fromitem.getName()
inside for loop.Result:
C:\projects\example\file1.txt C:\projects\example\file2.json C:\projects\example\file3.pdf