EN
Node.js - MySQL COUNT()
0
points
In this article, we would like to show you how to use SQL COUNT() function in Node.js.
Note: at the end of this article you can find database preparation SQL queries.
const mysql = require('mysql');
const connection = mysql.createConnection({ // gets connection with database
host: 'localhost', // '127.0.0.1'
user: 'root',
password: 'password',
database: 'my_database',
});
connection.connect(error => {
if (error) throw error;
const query ='SELECT COUNT(*) AS `number of users` FROM `users`';
connection.query(query, (error, result) => { // sends queries
connection.end(); // closes connection
if (error) throw error;
console.log(result);
});
});
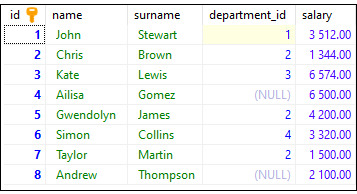
Output:
[ { 'number of users': 8 } ]
Database preparation
create_tables.sql
file:
CREATE TABLE `users` (
`id` INT(10) UNSIGNED NOT NULL AUTO_INCREMENT,
`name` VARCHAR(50) NOT NULL,
`surname` VARCHAR(50) NOT NULL,
`department_id` INT(10) UNSIGNED,
`salary` DECIMAL(15,2) NOT NULL,
PRIMARY KEY (`id`)
);
insert_data.sql
file:
INSERT INTO `users`
( `name`, `surname`, `department_id`, `salary`)
VALUES
('John', 'Stewart', 1, '3512.00'),
('Chris', 'Brown', 2, '1344.00'),
('Kate', 'Lewis', 3, '6574.00'),
('Ailisa', 'Gomez', NULL, '6500.00'),
('Gwendolyn', 'James', 2, '4200.00'),
('Simon', 'Collins', 4, '3320.00'),
('Taylor', 'Martin', 2, '1500.00'),
('Andrew', 'Thompson', NULL, '2100.00');