EN
Node.js - MySQL SELECT DISTINCT statement
0 points
In this article, we would like to show you how to make MySQL SELECT DISTINCT statement in Node.js.
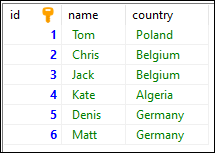
Note: at the end of this article you can find database preparation SQL queries.
xxxxxxxxxx
1
const mysql = require('mysql');
2
3
const connection = mysql.createConnection({ // gets connection with database
4
host: 'localhost', // '127.0.0.1'
5
user: 'root',
6
password: 'password',
7
database: 'database_name',
8
});
9
10
connection.connect(error => {
11
if (error) throw error;
12
const query ='SELECT DISTINCT `country` FROM `users`';
13
14
connection.query(query, (error, result) => { // sends queries
15
connection.end(); // closes connection
16
if (error) throw error;
17
console.table(result);
18
});
19
});
Result:
xxxxxxxxxx
1
┌─────────┬───────────┐
2
│ (index) │ country │
3
├─────────┼───────────┤
4
│ 0 │ 'Spain' │
5
│ 1 │ 'Italy' │
6
│ 2 │ 'Vietnam' │
7
│ 3 │ 'Poland' │
8
└─────────┴───────────┘
create_tables.sql
file:
xxxxxxxxxx
1
CREATE TABLE `users` (
2
`id` INT(10) UNSIGNED NOT NULL AUTO_INCREMENT,
3
`name` VARCHAR(100) NOT NULL,
4
`country` VARCHAR(15) NOT NULL,
5
PRIMARY KEY (`id`)
6
)
7
ENGINE=InnoDB;
insert_data.sql
file:
xxxxxxxxxx
1
INSERT INTO `users`
2
(`name`, `country`)
3
VALUES
4
('Tom', 'Poland'),
5
('Chris', 'Spain'),
6
('Jack', 'Spain'),
7
('Kim', 'Vietnam'),
8
('Marco', 'Italy'),
9
('Kate', 'Spain'),
10
('Nam', 'Vietnam');