EN
Node.js - MySQL FULL OUTER Join
0 points
In this article, we would like to show you how to use SQL FULL OUTER JOIN in Node.js.
Note: at the end of this article you can find database preparation SQL queries.
xxxxxxxxxx
1
const mysql = require('mysql');
2
3
const connection = mysql.createConnection({ // gets connection with database
4
host: 'localhost', // '127.0.0.1'
5
user: 'root',
6
password: 'password',
7
database: 'my_database',
8
});
9
10
connection.connect((error) => {
11
if (error) throw error;
12
const query = 'SELECT * ' +
13
'FROM `users` ' +
14
'LEFT JOIN `departments` ON `departments`.id = `users`.department_id ' +
15
'UNION ' +
16
'SELECT * ' +
17
'FROM `users` ' +
18
'RIGHT JOIN `departments` ON `departments`.id = `users`.department_id';
19
20
connection.query(query, (error, result) => { // sends queries
21
connection.end(); // closes connection
22
if (error) throw error;
23
console.log(result);
24
});
25
});
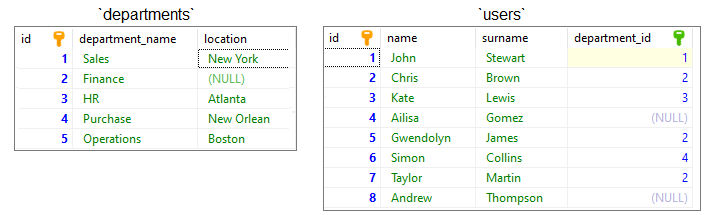
Output:
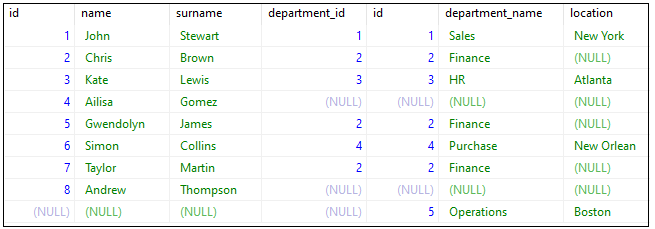
create_tables.sql
file:
xxxxxxxxxx
1
CREATE TABLE `departments` (
2
`id` INT(10) UNSIGNED NOT NULL,
3
`department_name` VARCHAR(50) NOT NULL,
4
`location` VARCHAR(50) NULL,
5
PRIMARY KEY (`id`)
6
);
7
8
CREATE TABLE `users` (
9
`id` INT(10) UNSIGNED NOT NULL AUTO_INCREMENT,
10
`name` VARCHAR(50) NOT NULL,
11
`surname` VARCHAR(50) NOT NULL,
12
`department_id` INT(10) UNSIGNED,
13
PRIMARY KEY (`id`),
14
FOREIGN KEY (`department_id`) REFERENCES `departments` (`id`)
15
)
insert_data.sql
file:
xxxxxxxxxx
1
INSERT INTO `departments`
2
(`id`, `department_name`, `location`)
3
VALUES
4
(1, 'Sales', 'New York'),
5
(2, 'Finance', NULL),
6
(3, 'HR', 'Atlanta'),
7
(4, 'Purchase', 'New Orlean'),
8
(5, 'Operations', 'Boston');
9
10
11
INSERT INTO `users`
12
( `name`, `surname`, `department_id`)
13
VALUES
14
('John', 'Stewart', 1),
15
('Chris', 'Brown', 2),
16
('Kate', 'Lewis', 3),
17
('Ailisa', 'Gomez', NULL),
18
('Gwendolyn', 'James', 2),
19
('Simon', 'Collins', 4),
20
('Taylor', 'Martin', 2),
21
('Andrew', 'Thompson', NULL);