EN
React - how to make left-side animated menu
3
points
Hey there! 👋 😊
In this post, I want to show you a left-side animated menu that I created recently.
Final effect:
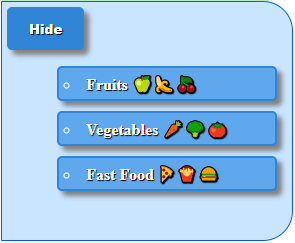
Below I present you how to create this simple customized left-side menu that displays list of different kind of food 🍒🥦🍟 on click event.
In the solution, I've used a modern approach that involves the use of functional components and React hooks. In this case useState
hook stores the state of my side menu, if it's visible or not.
There is also some styling in the example, which I recommend you carefully analyze and change as you want. You can modify the runnable example below and create your own left-side animated menu. 😊
Runnable example:
// ONLINE-RUNNER:browser;
// Note: Uncomment import lines during working with JSX Compiler.
// import React from 'react';
// import ReactDOM from 'react-dom';
const buttonStyle = {
padding: '10px 20px',
border: '2px solid #3085d6',
borderRadius: '5px',
background: '#3085d6',
boxShadow: '5px 5px 5px grey',
textShadow: '1px 1px 1px black',
fontWeight: '900',
color: 'white'
};
const commonStyle = {
position: 'fixed',
top: 0,
bottom: 0,
padding: '5px',
border: '1px solid #0657FF',
borderRadius: '0 30px 30px 0',
background: '#C9E5FF',
width: '280px',
transition: '0.5s',
overflow: 'hidden'
};
const visibleStyle = {
...commonStyle,
left: '0'
};
const hiddenStyle = {
...commonStyle,
left: '-300px'
};
const liStyle = {
margin: '10px',
padding: '5px',
border: '2px solid #3085d6',
borderRadius: '5px',
background: '#5fa8ed',
boxShadow: '5px 5px 5px grey',
textShadow: '1px 1px 1px black',
fontWeight: '900',
color: 'white',
listStyle: 'circle inside'
};
const App = () => {
const [visible, setVisible] = React.useState(false);
return (
<div style={{ height: "200px" }}>
<button style={buttonStyle} onClick={() => setVisible(true)}>
Show
</button>
<div style={visible ? visibleStyle : hiddenStyle}>
<button style={buttonStyle} onClick={() => setVisible(false)}>
Hide
</button>
<div>
<ul>
<li style={liStyle}>Fruits 🍏🍌🍒</li>
<li style={liStyle}>Vegetables 🥕🥦🍅</li>
<li style={liStyle}>Fast Food 🍕🍟🍔</li>
</ul>
</div>
</div>
</div>
);
};
const root = document.querySelector('#root');
ReactDOM.render(<App />, root );
Note:
To see the raw solution without unnecessary styling check out this article.