EN
How to create customized dynamic table in React
6
points
Hi everyone! 👋 😊
Last week I had a problem with creating a dynamic table in React, so I thought that maybe someone will find this solution helpful.
Effect of this short post:
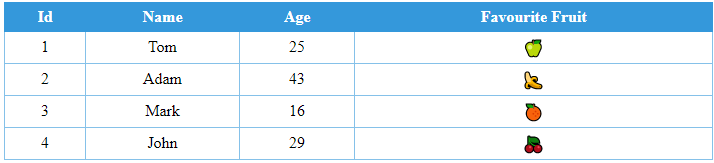
I took several steps in the solution below:
- I created a dynamic table based on array,
- Each table consists of a header and some data records,
- The header is fixed and keeps the same amount of columns,
- While creating records I usedÂ
map()
 function to convert array items into React elements.Â
Remember that each element should have a unique key 🗝ïžÂ because it helps React optimally manage changes in the DOM. Such a key may be for example theÂ
id
 assigned to each element of the table.
Runnable example:
// ONLINE-RUNNER:browser;
// Note: Uncomment import lines during working with JSX Compiler.
// import React from 'react';
// import ReactDOM from 'react-dom';
const tableStyle = {
border: '1px solid black',
borderCollapse: 'collapse',
textAlign: 'center',
width: '100%'
}
const tdStyle = {
border: '1px solid #85C1E9',
background: 'white',
padding: '5px'
};
const thStyle = {
border: '1px solid #3498DB',
background: '#3498DB',
color: 'white',
padding: '5px'
};
const App = () => {
const students = [
{ id: 1, name: 'Tom', age: 25, favFruit: '🍏' },
{ id: 2, name: 'Adam', age: 43, favFruit: '🍌' },
{ id: 3, name: 'Mark', age: 16, favFruit: '🍊' },
{ id: 4, name: 'John', age: 29, favFruit: '🍒' }
];
return (
<div>
<table style={tableStyle}>
<tbody>
<tr>
<th style={thStyle}>Id</th>
<th style={thStyle}>Name</th>
<th style={thStyle}>Age</th>
<th style={thStyle}>Favourite Fruit</th>
</tr>
{students.map(({ id, name, age, favFruit }) => (
<tr key={id}>
<td style={tdStyle}>{id}</td>
<td style={tdStyle}>{name}</td>
<td style={tdStyle}>{age}</td>
<td style={tdStyle}>{favFruit}</td>
</tr>
))}
</tbody>
</table>
</div>
);
};
const root = document.querySelector('#root');
ReactDOM.render(<App />, root );
Note:
Check out this article if you want to read more about dynamic tables. 😊