EN
MySQL - Alter Table
0
points
In this article, we would like to show you how to modify a table in MySQL.
Quick solution:
ALTER TABLE `table_name`
ADD `column_name` DATA_TYPE;
ALTER TABLE `table_name`
MODIFY `column_name` DATA_TYPE;
ALTER TABLE `table_name`
DROP `column_name`;
Note:
Go to the official documentation to see what you can use as
DATA_TYPE
.
Practical examples
To show how the ALTER TABLE
statement works, we will use the following table:
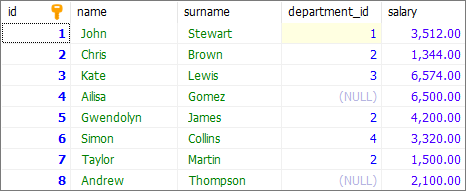
Note:
At the end of this article you can find database preparation SQL queries.
ALTER TABLE - ADD COLUMN
In this example, we will add email
column to the users
table.
Query:
ALTER TABLE `users`
ADD `email` VARCHAR(255);
Output (SELECT * FROM `users`
):
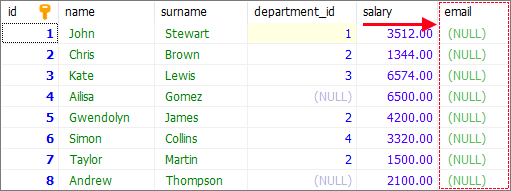
ALTER TABLE - DROP COLUMN
In this example, we will delete the department_id
column.
Query:
ALTER TABLE `users`
DROP `department_id`;
Output (SELECT * FROM `users`
):
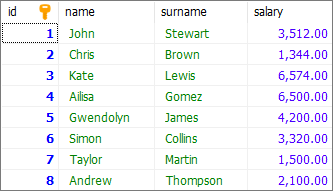
ALTER TABLE - MODIFY COLUMN
In this example, we will modify salary
column datatype from DECIMAL
to VARCHAR
.
Query:
ALTER TABLE `users`
MODIFY COLUMN `salary` VARCHAR(255);
Output:


Database preparation
create_tables.sql
file:
CREATE TABLE `users` (
`id` INT(10) UNSIGNED NOT NULL AUTO_INCREMENT,
`name` VARCHAR(50) NOT NULL,
`surname` VARCHAR(50) NOT NULL,
`department_id` INT(10) UNSIGNED,
`salary` DECIMAL(15,2) NOT NULL,
PRIMARY KEY (`id`)
);
insert_data.sql
file:
INSERT INTO `users`
( `name`, `surname`, `department_id`, `salary`)
VALUES
('John', 'Stewart', 1, '3512.00'),
('Chris', 'Brown', 2, '1344.00'),
('Kate', 'Lewis', 3, '6574.00'),
('Ailisa', 'Gomez', NULL, '6500.00'),
('Gwendolyn', 'James', 2, '4200.00'),
('Simon', 'Collins', 4, '3320.00'),
('Taylor', 'Martin', 2, '1500.00'),
('Andrew', 'Thompson', NULL, '2100.00');