EN
MySQL - CASE statement
3 points
In this article, we would like to show you how to use CASE
statement in MySQL.
Quick solution:
xxxxxxxxxx
1
CASE
2
WHEN condition1 THEN result1
3
WHEN condition2 THEN result2
4
WHEN conditionN THEN resultN
5
ELSE result
6
END;
To show how the CASE
statement works, we will use the following table:
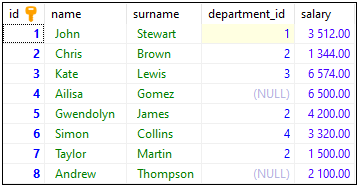
Note:
At the end of this article you can find database preparation SQL queries.
In this example, we will display information about users salary using CASE
statement.
Query:
xxxxxxxxxx
1
SELECT `name`, `salary`,
2
CASE
3
WHEN `salary` > 6000 THEN 'Salary greater than 6000$'
4
WHEN `salary` > 4000 THEN 'Salary greater than 4000$'
5
WHEN `salary` >= 2000 THEN 'Salary greater than 2000$'
6
ELSE 'Salary less than 2000$'
7
END AS 'Salary information'
8
FROM `users`;
Output:
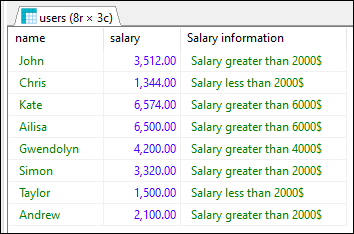
create_tables.sql
file:
xxxxxxxxxx
1
CREATE TABLE `users` (
2
`id` INT(10) UNSIGNED NOT NULL AUTO_INCREMENT,
3
`name` VARCHAR(50) NOT NULL,
4
`surname` VARCHAR(50) NOT NULL,
5
`department_id` INT(10) UNSIGNED,
6
`salary` DECIMAL(15,2) NOT NULL,
7
PRIMARY KEY (`id`)
8
);
insert_data.sql
file:
xxxxxxxxxx
1
INSERT INTO `users`
2
( `name`, `surname`, `department_id`, `salary`)
3
VALUES
4
('John', 'Stewart', 1, '3512.00'),
5
('Chris', 'Brown', 2, '1344.00'),
6
('Kate', 'Lewis', 3, '6574.00'),
7
('Ailisa', 'Gomez', NULL, '6500.00'),
8
('Gwendolyn', 'James', 2, '4200.00'),
9
('Simon', 'Collins', 4, '3320.00'),
10
('Taylor', 'Martin', 2, '1500.00'),
11
('Andrew', 'Thompson', NULL, '2100.00');