EN
MySQL - Create or replace view
0 points
In this article, we would like to show you how to create and update a view using CREATE OR REPLACE VIEW
in MySQL.
Quick solution:
xxxxxxxxxx
1
CREATE OR REPLACE VIEW `view_name` AS
2
SELECT `column1`, `column2`, `columnN`
3
FROM `table_name`
4
WHERE condition;
To show how to create or replace a view, we will use the following table:
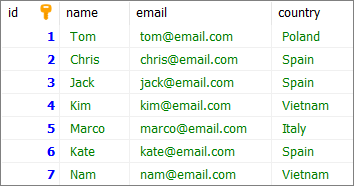
Note:
At the end of this article you can find database preparation SQL queries.
In this example, we will create a view using CREATE OR REPLACE VIEW
statement.
Query:
xxxxxxxxxx
1
CREATE OR REPLACE VIEW `spain_users` AS
2
SELECT *
3
FROM `users`
4
WHERE `country` = 'Spain';
Result:
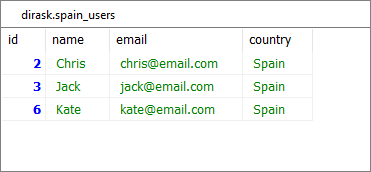
In this example, we will remove the country
column from the view created in Example 1. We will remove the column by updating the existing view using CREATE OR REPLACE VIEW
statement.
Query:
xxxxxxxxxx
1
CREATE OR REPLACE VIEW `spain_users` AS
2
SELECT `id`, `name`, `email`
3
FROM `users`
4
WHERE `country` = 'Spain';
Result:
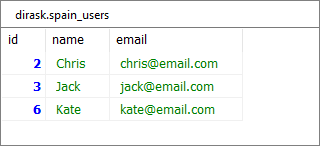
create_tables.sql
file:
xxxxxxxxxx
1
CREATE TABLE `users` (
2
`id` INT(10) UNSIGNED NOT NULL AUTO_INCREMENT,
3
`name` VARCHAR(100) NOT NULL,
4
`email` VARCHAR(100) NOT NULL,
5
`country` VARCHAR(15) NOT NULL,
6
PRIMARY KEY (`id`)
7
)
8
ENGINE=InnoDB;
insert_data.sql
file:
xxxxxxxxxx
1
INSERT INTO `users`
2
(`name`, `email`, `country`)
3
VALUES
4
('Tom', 'tom@email.com', 'Poland'),
5
('Chris','chris@email.com', 'Spain'),
6
('Jack','jack@email.com', 'Spain'),
7
('Kim','kim@email.com', 'Vietnam'),
8
('Marco','marco@email.com', 'Italy'),
9
('Kate','kate@email.com', 'Spain'),
10
('Nam','nam@email.com', 'Vietnam');
create_view.sql
file:
xxxxxxxxxx
1
CREATE VIEW `spain_users` AS
2
SELECT *
3
FROM `users`
4
WHERE `country` = 'Spain';