EN
JavaScript - draw function chart on canvas element
10 points
In this short article, we would like to show a simple way how to plot a chart on HTML5 canvas element using JavaScript.
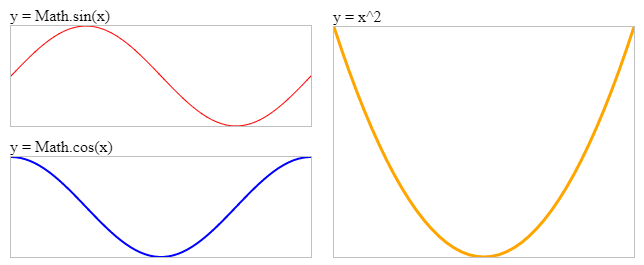
Practical example:
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style> canvas { border: 1px solid silver; } </style>
5
</head>
6
<body>
7
<div><div>y = Math.sin(x)</div><canvas id="sin-canvas" width="400" height="130"></canvas></div>
8
<div><div>y = Math.cos(x)</div><canvas id="cos-canvas" width="400" height="130"></canvas></div>
9
<div><div>y = x^2 </div><canvas id="x2-canvas" width="400" height="250"></canvas></div>
10
<script>
11
12
function Calculator(canvas, x1, x2, y1, y2, func) {
13
var cWidth = canvas.width;
14
var cHeight = canvas.height;
15
var xRange = x2 - x1;
16
var yRange = y2 - y1;
17
this.calculatePoint = function(x) {
18
var y = func(x);
19
// chart will be reversed horizontaly because of reversed canvas pixels
20
var nx = (x - x1) / xRange; // normalized x
21
var ny = 1.0 - (y - y1) / yRange; // normalized y
22
return {
23
x: nx * cWidth,
24
y: ny * cHeight
25
};
26
};
27
}
28
29
function Chart(canvas, x1, x2, y1, y2, dx, func) {
30
var context = canvas.getContext('2d');
31
var calculator = new Calculator(canvas, x1, x2, y1, y2, func);
32
this.drawChart = function(color, width) {
33
var a = calculator.calculatePoint(x1);
34
var b = calculator.calculatePoint(x2);
35
context.beginPath();
36
context.moveTo(a.x, a.y);
37
for (var x = x1 + dx; x < x2; x += dx) {
38
var point = calculator.calculatePoint(x);
39
context.lineTo(point.x, point.y);
40
}
41
context.lineTo(b.x, b.y);
42
context.strokeStyle = color || 'black';
43
context.lineWidth = width || 1;
44
context.stroke();
45
};
46
}
47
48
49
// Usage example:
50
51
var sinCanvas = document.querySelector('#sin-canvas');
52
var cosCanvas = document.querySelector('#cos-canvas');
53
var x2Canvas = document.querySelector('#x2-canvas');
54
55
var dx = 0.1; // x value step
56
57
var sinChart = new Chart(sinCanvas, 0, 2 * Math.PI, -1, +1, dx, Math.sin); // x: <0, 360> degress
58
var cosChart = new Chart(cosCanvas, 0, 2 * Math.PI, -1, +1, dx, Math.cos); // x: <0, 360> degress
59
var x2Chart = new Chart(x2Canvas, -2, +2, 0, 4, dx, function(x) { return x * x });
60
61
sinChart.drawChart('red', 1);
62
cosChart.drawChart('blue', 2);
63
x2Chart.drawChart('orange', 3);
64
65
</script>
66
</body>
67
</html>