EN
JavaScript - draw integral on canvas element
4 points
In this short article, we would like to show a simple way how to draw integral function on HTML5 canvas element using JavaScript.
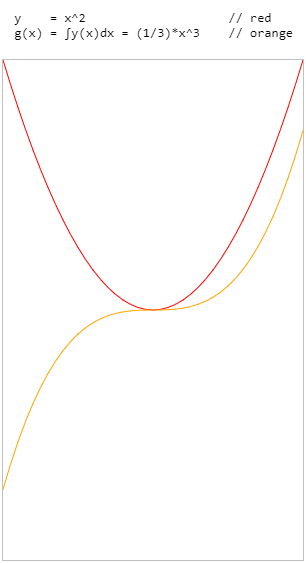
To draw function and integral function we should use the following formula for calculations:
xxxxxxxxxx
1
const dx = 0.2; // x value step - used only for calculations precision
2
// if dx is very small then calculations are more precised
3
4
const x2Function = x => x * x;
5
6
// Integral will be calculated on <x1, x2> range.
7
8
const x1 = -2;
9
const x2 = +2;
10
11
const zeroValue = (x1 < 0 && x2 > 0 ? dx * x2Function(0) : 0);
12
13
let negativeSum = 0;
14
let positiveSum = 0;
15
16
const calculateX2NegativeIntegral = x => (negativeSum -= dx * x2Function(x));
17
const calculateX2PositiveIntegral = x => (positiveSum += dx * x2Function(x));
18
19
console.log(`Negative part:`);
20
21
for (let x = 0; x > x1; x -= dx) { // it is very important to iterate from 0 to x1
22
console.log(`(${x}, ${calculateX2NegativeIntegral(x)})`);
23
}
24
25
console.log(`Positive part:`);
26
27
for (let x = 0; x < x2; x += dx) { // it is very important to iterate from 0 to x2
28
console.log(`(${x}, ${calculateX2PositiveIntegral(x)})`);
29
}
Practical example:
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style> canvas { border: 1px solid silver; } </style>
5
</head>
6
<body>
7
<pre>
8
y = x^2 // red
9
g(x) = ∫y(x)dx = (1/3)*x^3 // orange
10
</pre>
11
<canvas id="canvas" width="300" height="500"></canvas>
12
<script>
13
14
function iterateNegative(x1, x2, dx, callback) {
15
for (var x = x1 + dx; x > x2; x += dx) {
16
callback(x);
17
}
18
return x1 > x2;
19
}
20
21
function iteratePositive(x1, x2, dx, callback) {
22
for (var x = x1 + dx; x < x2; x += dx) {
23
callback(x);
24
}
25
return x1 < x2;
26
}
27
28
function Calculator(canvas, x1, x2, y1, y2, func) {
29
var cWidth = canvas.width;
30
var cHeight = canvas.height;
31
var xRange = x2 - x1;
32
var yRange = y2 - y1;
33
this.calculatePoint = function(x) {
34
var y = func(x);
35
// chart will be reversed horizontaly because of reversed canvas pixels
36
var nx = (x - x1) / xRange; // normalized x
37
var ny = 1.0 - (y - y1) / yRange; // normalized y
38
return {
39
x: nx * cWidth,
40
y: ny * cHeight
41
};
42
};
43
}
44
45
function Chart(canvas, calculator, iterate) {
46
var context = canvas.getContext('2d');
47
var callback = function(x) {
48
var point = calculator.calculatePoint(x);
49
context.lineTo(point.x, point.y);
50
};
51
this.drawChart = function(color, width, x1, x2, dx) {
52
var a = calculator.calculatePoint(x1);
53
context.beginPath();
54
context.moveTo(a.x, a.y);
55
if (iterate(x1, x2, dx, callback)) {
56
var b = calculator.calculatePoint(x2);
57
context.lineTo(b.x, b.y);
58
}
59
context.strokeStyle = color || 'black';
60
context.lineWidth = width || 1;
61
context.stroke();
62
};
63
};
64
65
function FunctionChart(canvas, x1, x2, y1, y2, dx, func) {
66
var calculator = new Calculator(canvas, x1, x2, y1, y2, func);
67
var chart = new Chart(canvas, calculator, iteratePositive);
68
this.drawChart = function(color, width) {
69
chart.drawChart(color, width, x1, x2, dx);
70
};
71
}
72
73
function IntegralChart(canvas, x1, x2, y1, y2, dx, c, func) {
74
var zeroValue = (x1 < 0 && x2 > 0 ? dx * func(0) : 0);
75
var negativeSum = zeroValue + c;
76
var positiveSum = zeroValue + c;
77
var negativeIntegral = function(x) {
78
if (x < 0) {
79
negativeSum -= dx * func(x);
80
}
81
return negativeSum;
82
};
83
var positiveIntegral = function(x) {
84
if (x > 0) {
85
positiveSum += dx * func(x);
86
}
87
return positiveSum;
88
};
89
var negativeCalculator = new Calculator(canvas, x1, x2, y1, y2, negativeIntegral);
90
var positiveCalculator = new Calculator(canvas, x1, x2, y1, y2, positiveIntegral);
91
var negativeChart = new Chart(canvas, negativeCalculator, iterateNegative);
92
var positiveChart = new Chart(canvas, positiveCalculator, iteratePositive);
93
this.drawChart = function(color, width) {
94
negativeSum = zeroValue + c;
95
positiveSum = zeroValue + c;
96
negativeChart.drawChart(color, width, 0, x1, -dx);
97
positiveChart.drawChart(color, width, 0, x2, +dx);
98
};
99
};
100
101
102
// Usage example:
103
104
var canvas = document.querySelector('#canvas');
105
106
var dx = 0.1; // x value step used to calculate derivate
107
var c = 0.0; // constant (y shift of integral function)
108
109
var x2Function = function(x) {
110
return x * x;
111
};
112
113
var functionChart = new FunctionChart(canvas, -2, +2, -4, 4, dx, x2Function);
114
var integralChart = new IntegralChart(canvas, -2, +2, -4, 4, dx, c, x2Function);
115
116
functionChart.drawChart('red', 1);
117
integralChart.drawChart('orange', 1);
118
119
</script>
120
</body>
121
</html>