EN
JavaScript - draw derivative on canvas element
9 points
In this short article, we would like to show a simple way how to draw derivative function on HTML5 canvas element using JavaScript.
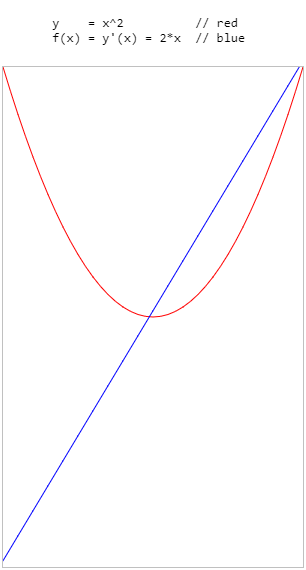
To draw function and derivate function we should use the following formula for calculations:
xxxxxxxxxx
1
var dx = 0.1; // x value step - used only for calculations precision
2
// if dx is very small then calculations are more precised
3
4
var x2Function = function(x) {
5
return x * x;
6
};
7
8
// Dervitive function from definition:
9
// lim (dx->0) (f(x + dx) - f(x)) / dx or: lim (h->0) (f(x + h) - f(x)) / h
10
//
11
// https://en.wikipedia.org/wiki/Derivative#Definition
12
//
13
var x2Derivative = function(x) {
14
var dy = x2Function(x + dx) - x2Function(x);
15
return dy / dx;
16
};
Practical example:
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style> canvas { border: 1px solid silver; } </style>
5
</head>
6
<body>
7
<pre>
8
y = x^2 // red
9
f(x) = y'(x) = 2*x // blue
10
</pre>
11
<canvas id="canvas" width="300" height="500"></canvas>
12
<script>
13
14
function Calculator(canvas, x1, x2, y1, y2, func) {
15
var cWidth = canvas.width;
16
var cHeight = canvas.height;
17
var xRange = x2 - x1;
18
var yRange = y2 - y1;
19
this.calculatePoint = function(x) {
20
var y = func(x);
21
// chart will be reversed horizontaly because of reversed canvas pixels
22
var nx = (x - x1) / xRange; // normalized x
23
var ny = 1.0 - (y - y1) / yRange; // normalized y
24
return {
25
x: nx * cWidth,
26
y: ny * cHeight
27
};
28
};
29
}
30
31
function FunctionChart(canvas, x1, x2, y1, y2, dx, func) {
32
var context = canvas.getContext('2d');
33
var calculator = new Calculator(canvas, x1, x2, y1, y2, func);
34
this.drawChart = function(color, width) {
35
var a = calculator.calculatePoint(x1);
36
var b = calculator.calculatePoint(x2);
37
context.beginPath();
38
context.moveTo(a.x, a.y);
39
for (var x = x1 + dx; x < x2; x += dx) {
40
var point = calculator.calculatePoint(x);
41
context.lineTo(point.x, point.y);
42
}
43
context.lineTo(b.x, b.y);
44
context.strokeStyle = color || 'black';
45
context.lineWidth = width || 1;
46
context.stroke();
47
};
48
}
49
50
function DerivativeChart(canvas, x1, x2, y1, y2, dx, func) {
51
var derivative = function(x) {
52
var dy = func(x + dx) - func(x);
53
return dy / dx;
54
};
55
var chart = new FunctionChart(canvas, x1, x2, y1, y2, dx, derivative);
56
this.drawChart = function(color, width) {
57
chart.drawChart(color, width);
58
};
59
}
60
61
62
// Usage example:
63
64
var canvas = document.querySelector('#canvas');
65
66
var dx = 0.1; // x value step used to calculate integral
67
68
var x2Function = function(x) {
69
return x * x;
70
};
71
72
var functionChart = new FunctionChart(canvas, -2, +2, -4, 4, dx, x2Function);
73
var derivativeChart = new DerivativeChart(canvas, -2, +2, -4, 4, dx, x2Function);
74
75
functionChart.drawChart('red', 1);
76
derivativeChart.drawChart('blue', 1);
77
78
</script>
79
</body>
80
</html>