EN
Node.js - MySQL HAVING clause
0
points
In this article, we would like to show you how to use MySQLΒ HAVINGΒ in Node.js.
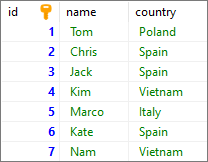
Note:Β at the end of this article you can find database preparation SQL queries.
const mysql = require('mysql');
const connection = mysql.createConnection({ // gets connection with database
host: 'localhost', // '127.0.0.1'
user: 'root',
password: 'password',
database: 'database_name',
});
connection.connect(error => {
if (error) throw error;
const query ='SELECT COUNT(`id`) AS `number of users`, `country` ' +
'FROM `users` ' +
'GROUP BY `country` ' +
'HAVING COUNT(`id`) >= ?;';
const minimum = [2]; // Each group will have at least 2 users.
connection.query(query, minimum, (error, result) => { // sends queries
connection.end(); // closes connection
if (error) throw error;
console.table(result);
});
});
Result:Β
βββββββββββ¬ββββββββββββββββββ¬ββββββββββββ
β (index) β number of users β country β
βββββββββββΌββββββββββββββββββΌββββββββββββ€
β 0 β '3' β 'Spain' β
β 1 β '2' β 'Vietnam' β
βββββββββββ΄ββββββββββββββββββ΄ββββββββββββ
Database preparation
create_tables.sql
Β file:
CREATE TABLE `users` (
`id` INT(10) UNSIGNED NOT NULL AUTO_INCREMENT,
`name` VARCHAR(100) NOT NULL,
`country` VARCHAR(15) NOT NULL,
PRIMARY KEY (`id`)
)
ENGINE=InnoDB;
insert_data.sql
Β file:
INSERT INTO `users`
(`name`, `country`)
VALUES
('Tom', 'Poland'),
('Chris', 'Spain'),
('Jack', 'Spain'),
('Kim', 'Vietnam'),
('Marco', 'Italy'),
('Kate', 'Spain'),
('Nam', 'Vietnam');