EN
VS Code - debug Express.js under Node.js server
5 points
In this short article, we would like to show how to configure VS Code to debug Express.js applications.
Quick solution (create the following .vscode/launch.json
configuration file):
xxxxxxxxxx
1
{
2
"version": "0.2.0",
3
"configurations": [
4
{
5
"command": "node index.js",
6
"name": "My Backend",
7
"request": "launch",
8
"type": "node-terminal"
9
}
10
]
11
}
Hint: you can change
"node index.js"
to"nodemon index.js
" to debug Expess.js withnodemon
.
Screenshot:
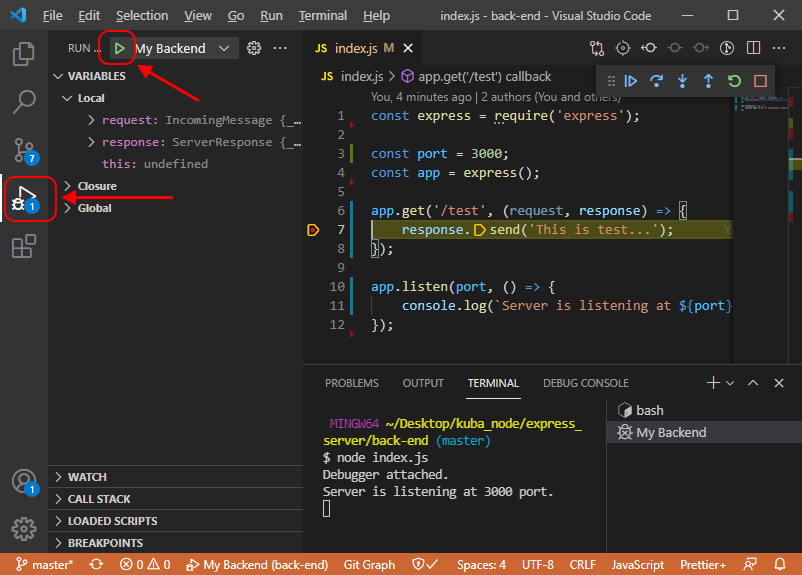
xxxxxxxxxx
1
/C/
2
|
3
+-- back-end/
4
|
5
+-- .vscode/
6
| |
7
| +-- launch.json
8
|
9
+-- node_modules/
10
+-- index.js
11
+-- package-lock.json
12
+-- package.json
index.js
file:
xxxxxxxxxx
1
const express = require('express');
2
3
const port = 3000;
4
const app = express();
5
6
app.get('/test', (request, response) => {
7
response.send('This is test...');
8
});
9
10
app.listen(port, () => {
11
console.log(`Server is listening at ${port} port.`);
12
});
package.json
file:
xxxxxxxxxx
1
{
2
"name": "back-end",
3
"version": "1.0.0",
4
"description": "",
5
"main": "index.js",
6
"scripts": {
7
"test": "echo \"Error: no test specified\" && exit 1",
8
"start": "node index.js",
9
"dev": "nodemon index.js"
10
},
11
"keywords": [],
12
"author": "",
13
"license": "ISC",
14
"dependencies": {
15
"express": "^4.17.1",
16
"nodemon": "^2.0.12"
17
}
18
}
Node.js packages should be installed with the following command:
xxxxxxxxxx
1
npm ci install
Where: ci
parameter runs installation without changing the version of the used packages.