EN
Create simple animated expander in React
6
points
Hi there! 👋 😊
Today, I was thinking about creating an animated expander in React and I came up with the following solution.
Effect of this short post:
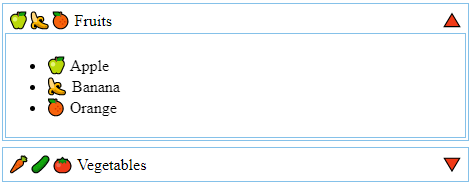
In the example below, I create a simple expander that displays Fruits 🍉 and Vegetables 🍅 lists on click event. I've used a modern approach that involves the use of functional components and React hooks. In this case useState
hook stores the state of my expander. 🔺🔻
Runnable example:
// ONLINE-RUNNER:browser;
//Note: Uncomment import lines during working with JSX Compiler.
// import React from 'react';
// import ReactDOM from 'react-dom';
const expanderStyle = {
margin: '6px 0',
padding: '2px',
border: '1px solid #85C1E9'
};
const headerStyle = {
display: 'flex',
cursor: 'pointer'
};
const titleStyle = {
padding: '3px',
flex: 'none'
};
const spacerStyle = {
flex: '1'
};
const iconStyle = {
padding: '3px',
flex: 'none'
};
const contentStyle = {
overflow: 'hidden',
transition: 'all 0.3s'
};
const contentExpandedStyle = {
...contentStyle,
padding: '4px 0',
border: '1px solid #85C1E9',
height: 'auto',
filter: 'opacity(1)'
};
const contentCollapsedStyle = {
...contentStyle,
padding: '0 0',
border: '1px solid transparent',
height: '0',
filter: 'opacity(0)'
};
const Expander = ({title, children}) => {
const [expanded, setExpanded] = React.useState(false);
const handleHeaderClick = () => {
setExpanded(expanded => !expanded);
};
return (
<div style={expanderStyle}>
<div style={headerStyle} onClick={handleHeaderClick}>
<div style={titleStyle}>{title}</div>
<div style={spacerStyle} />
<div style={iconStyle}>{expanded ? '🔺' : '🔻'}</div>
</div>
<div style={expanded ? contentExpandedStyle : contentCollapsedStyle}>
{children}
</div>
</div>
);
};
// Usage example:
const App = () => {
return (
<div style={{height: '260px'}}>
<Expander title="🍏🍌🍊 Fruits">
<ul>
<li>🍏 Apple</li>
<li>🍌 Banana</li>
<li>🍊 Orange</li>
</ul>
</Expander>
<Expander title="🥕🥒🍅 Vegetables">
<ul>
<li>🥕 Carrot</li>
<li>🥒 Cucumber</li>
<li>🍅 Tomato</li>
</ul>
</Expander>
</div >
);
};
const root = document.querySelector('#root');
ReactDOM.render(<App/>, root );