EN
JavaScript - draw image on canvas element
3
points
Quick solution:
// drawing image at position 50x50 px with size 140x140 px
context.drawImage(image, 50, 50, 140, 140);
Using JavaScript canvas element context it is possible to draw image in following way.
1. drawImage()
method example
With this approach HTMLImageElement
type handle is necessary.
Avaialble approaches to get element handle are:
- with
document.querySelector()
,docuemnt.getElementById()
or other similar method, - with
document.createElement('img')
execution, - with
new Image()
execution.
Following example shows how to use new Image()
approach:
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<style>
#my-canvas { border: 1px solid gray; }
</style>
</head>
<body>
<canvas id="my-canvas" width="200" height="200"></canvas>
<script>
var canvas = document.querySelector('#my-canvas');
var context = canvas.getContext('2d');
var image = new Image();
image.onload = function() {
context.drawImage(image, 50, 50, 140, 140);
};
image.onerror = function() {
context.fillStyle = 'red';
context.font = '16px Arial';
context.fillText('Image loading error!', 10, 30);
};
image.src = 'https://dirask.com/static/bucket/1574890428058-BZOQxN2D3p--image.png';
</script>
</body>
</html>
Used resources:
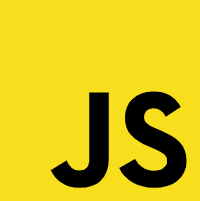