EN
JavaScript - get only certain part of canvas
0
points
In this article, we would like to show you how to get only a certain part of canvas using JavaScript
Quick solution:
var canvas = document.querySelector('#canvas-id');
var context = canvas.getContext('2d');
context.drawImage(image, dx, dy);
or:
var canvas = document.querySelector('#canvas-id');
var context = canvas.getContext('2d');
context.drawImage(image, sx, sy, sWidth, sHeight, dx, dy, dWidth, dHeight);
Practical examples
1. Using drawImage()
with three arguments
In this example, we use drawImage()
method with three arguments so we can get part of the canvas and place its upper-left corner into the dx
, dy
coordinates.
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<style>
#my-canvas { border: 1px solid gray; }
</style>
</head>
<body>
<img id="image" src="https://dirask.com/static/bucket/1633539280748-ymAGm6LYq6--image.png" />
<br>
<canvas id="my-canvas" width="300" height="300"></canvas>
<script>
var canvas = document.querySelector('#my-canvas');
var context = canvas.getContext('2d');
var image = document.querySelector('#image');
image.onload = function() {
context.drawImage(image, 50, 100); // drawImage(image, dx, dy)
};
image.onerror = function() {
context.fillStyle = 'red';
context.font = '16px Arial';
context.fillText('Image loading error!', 10, 30);
};
</script>
</body>
</html>
Result:
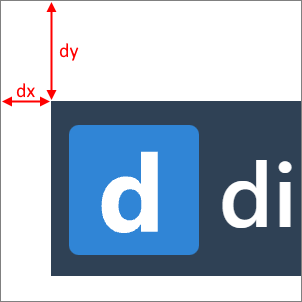
3. Using drawImage()
with nine arguments
With this approach, we are able to specify the parameters of the part that we want to get, where we want to place it and its size on the destination canvas.
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<style>
#my-canvas { border: 1px solid gray; }
</style>
</head>
<body>
<img id="image" src="https://dirask.com/static/bucket/1633539280748-ymAGm6LYq6--image.png" />
<br>
<canvas id="my-canvas" width="300" height="300"></canvas>
<script>
var canvas = document.querySelector('#my-canvas');
var context = canvas.getContext('2d');
var image = document.querySelector('#image'); // 440px x 175px
image.onload = function() {
context.drawImage(image, 20, 25, 110, 120, 50, 80, 110, 120);
};
image.onerror = function() {
context.fillStyle = 'red';
context.font = '16px Arial';
context.fillText('Image loading error!', 10, 30);
};
</script>
</body>
</html>
Result:
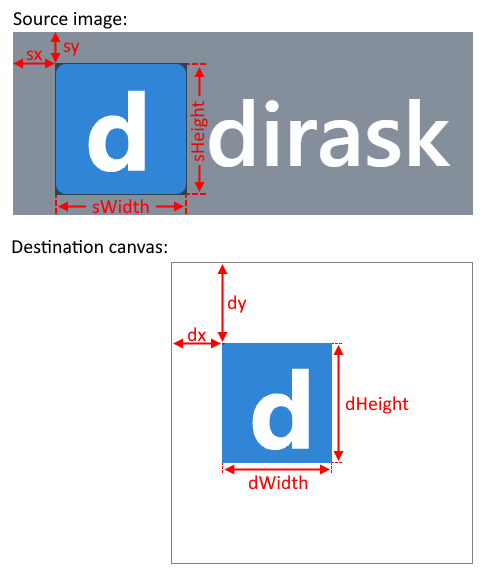