EN
Java - read all text from file with custom FileUtils
11
points
In this article, we would like to show how in Java, read all text from file to string.
Quick solution:
package com.dirask.examples;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.Reader;
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
class Program {
public static void main(String[] args) throws IOException {
String path = "C:\\Project\\input.txt";
String text = readText(path, StandardCharsets.UTF_8);
System.out.println(text);
}
private static String readText(String path, Charset charset) throws IOException {
StringBuilder builder = new StringBuilder();
try (FileInputStream stream = new FileInputStream(path);
Reader reader = new InputStreamReader(stream, charset))
{
char[] buffer = new char[1024];
while (true) {
int count = reader.read(buffer, 0, buffer.length);
if (count == -1) {
break;
}
builder.append(buffer, 0, count);
}
}
return builder.toString();
}
}
Example output:
This is 1st line...
This is 2nd line...
This is 3rd line...
Example input.txt
:
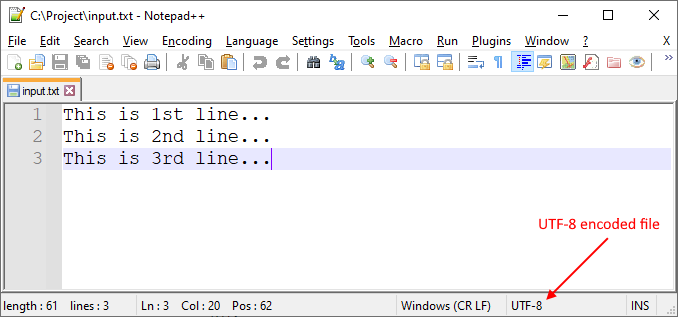
This article presents some simple util that lets users to read file using: String
path, File
object, Path
object, Reader
object or InputStream
object.
1. File reading example
Note: check below
FileUtils.java
file to see how text reading util looks.
Program.java
file:
package com.dirask.examples;
import java.io.*;
import java.nio.file.Paths;
public class Program {
public static void main(String[] args) throws IOException {
readWithStringPath();
readWithFileObject();
readWithPathObject();
readWithReaderObject();
readWithInputStreamObject();
}
private static void readWithStringPath() {
String text = FileUtils.readText("C:\\Project\\input.txt");
System.out.println(text);
}
private static void readWithFileObject() {
String text = FileUtils.readText(new File("C:\\Project\\input.txt"));
System.out.println(text);
}
private static void readWithPathObject() {
String text = FileUtils.readText(Paths.get("C:\\Project\\input.txt"));
System.out.println(text);
}
private static void readWithReaderObject() {
try(FileReader reader = new FileReader("C:\\Project\\input.txt")) {
String text = FileUtils.readText(reader);
System.out.println(text);
}
}
private static void readWithInputStreamObject() {
try(InputStream stream = new FileInputStream("C:\\Project\\input.txt")) {
String text = FileUtils.readText(stream);
System.out.println(text);
}
}
}
2. Used file utils
FileUtils.java
file:
package com.dirask.examples;
import java.io.*;
import java.nio.file.Path;
public class FileUtils {
public static String readText(Reader reader) throws IOException {
StringBuilder builder = new StringBuilder();
char[] buffer = new char[1024];
while (true) {
int count = reader.read(buffer, 0, buffer.length);
if (count == -1) {
break;
}
builder.append(buffer, 0, count);
}
return builder.toString();
}
public static String readText(InputStream stream) throws IOException {
Reader reader = new InputStreamReader(stream, "UTF-8");
return readText(reader);
}
public static String readText(File file) throws IOException {
try (InputStream stream = new FileInputStream(file)) {
return readText(stream);
}
}
public static String readText(Path path) throws IOException {
File file = path.toFile();
return readText(file);
}
public static String readText(String path) throws IOException {
try (FileInputStream stream = new FileInputStream(path)) {
return readText(stream);
}
}
}
3. Used input resources
input.txt
file (full path is C:\Project\input.txt
):
This is 1st line...
This is 2nd line...
This is 3rd line...
Merged questions
- Java - how to read all lines as one string from file object?
- Java - how to read all lines as one string from input stream object?
- Java - how to read all lines as one string from reader object?
- Java - how to read all lines as one string using file path string?
- Java - how to read all lines as one string using path object?
See also
Alternative titles
- Java - how to read all lines as one string from file object?
- Java - how to read all lines as one string from input stream object?
- Java - how to read all lines as one string from reader object?
- Java - how to read all lines as one string using file path string?
- Java - how to read all lines as one string using path object?