EN
Java read text from file (simple way)
3
points
Solution 1
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.List;
public class ReadTextExample {
public static void main(String[] args) throws IOException {
Path path = Paths.get("C:\\projects\\text_file.txt");
List<String> lines = Files.readAllLines(path, StandardCharsets.UTF_8);
for (String line : lines) {
System.out.println(line);
}
}
}
Output:
line 1
line 2
line 3
Solution 2
We can also read entire text file in just 1 line of code in java:
new String(Files.readAllBytes(path))
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class ReadTextExample {
public static void main(String[] args) throws IOException {
Path path = Paths.get("C:\\projects\\text_file.txt");
String entireTextFile = new String(Files.readAllBytes(path));
System.out.println(entireTextFile);
}
}
Output:
line 1
line 2
line 3
Solution 3
import java.io.*;
import java.nio.charset.StandardCharsets;
import java.nio.file.Path;
import java.nio.file.Paths;
public class FileUtils {
public static String readText(Path path) throws IOException {
File file = path.toFile();
StringBuilder builder = new StringBuilder();
char[] buffer = new char[1024];
try (InputStream stream = new FileInputStream(file);
Reader reader = new InputStreamReader(stream, StandardCharsets.UTF_8)
) {
while (true) {
int count = reader.read(buffer, 0, buffer.length);
if (count == -1)
break;
builder.append(buffer, 0, count);
}
}
return builder.toString();
}
// usage example:
public static void main(String[] args) throws IOException {
String text = FileUtils.readText(Paths.get("C:\\projects\\text_file.txt"));
System.out.println(text);
}
}
Output:
line 1
line 2
line 3
Input data
Input data preparation for examples in this article.
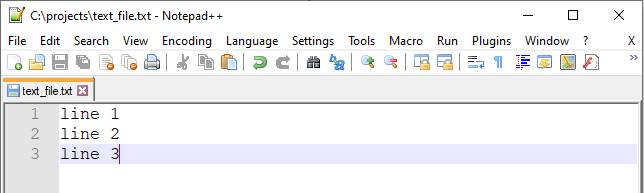
File path under Windows operating system:
C:\\projects\\text_file.txt
File content:
line 1
line 2
line 3