EN
JavaScript - stopwatch with split time feature
13 points
In this article, we would like to show you how to make a stopwatch with a lap time feature in JavaScript.
This section shows how to write a custom stopwatch.
Screenshots:
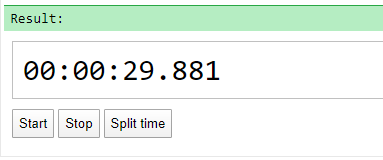
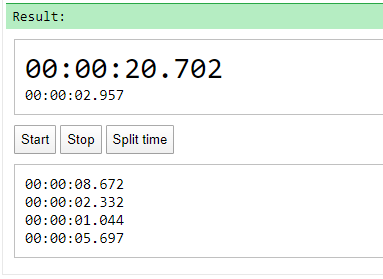
In the below example, we've created 3 buttons - Start, Stop, Split time. Play with this example to see how it works.
Runnable example:
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
6
#display, #times {
7
margin: 10px 0px;
8
padding: 10px;
9
border: 1px solid silver;
10
font-family: monospace;
11
}
12
13
.total-time { /* total measured time */
14
font-size: 30px;
15
}
16
17
.split-time { /* time of last split */
18
font-size: 15px;
19
}
20
21
button {
22
margin: 0px;
23
padding: 5px;
24
}
25
26
</style>
27
<script>
28
29
function calculatePeriod(t1, t2) {
30
var dt = t2 - t1;
31
32
var units = [
33
{name: 'milliseconds', scale: 1000},
34
{name: 'seconds', scale: 60},
35
{name: 'minutes', scale: 60},
36
{name: 'hours', scale: 24}
37
];
38
39
var result = { };
40
41
for(var i = 0; i < units.length; ++i) {
42
var unit = units[i];
43
44
var total = Math.floor(dt / unit.scale);
45
var rest = dt - total * unit.scale;
46
47
result[unit.name] = rest;
48
49
dt = total;
50
}
51
52
result.days = dt;
53
54
return result;
55
}
56
57
function padLeft(number, length, character) {
58
if(character == null)
59
character = '0';
60
61
var result = number.toString();
62
63
for(var i = result.length; i < length; ++i) {
64
result = character + result;
65
}
66
67
return result;
68
}
69
70
function renderTime(t1, t2) {
71
var period = calculatePeriod(t1, t2);
72
73
var text = '';
74
75
if (period.days) {
76
text += padLeft(period.days, 2) + ' days ';
77
}
78
79
text += padLeft(period.hours, 2) + ':';
80
text += padLeft(period.minutes, 2) + ':';
81
text += padLeft(period.seconds, 2) + '.';
82
text += padLeft(period.milliseconds, 3);
83
84
return text;
85
}
86
87
</script>
88
</head>
89
<body>
90
<div id="display">
91
<div class="total-time">
92
00:00:00.000
93
</div>
94
</div>
95
<button onclick="startStopwatch();">Start</button>
96
<button onclick="stopStopwatch();">Stop</button>
97
<button onclick="splitTime();">Split time</button>
98
<div id="times" style="display: none;"></div>
99
<script>
100
101
var interval = null; // interval id
102
103
var start = null; // start time
104
var split = null; // split time
105
106
var display = document.getElementById('display');
107
var times = document.getElementById('times');
108
109
function startStopwatch() {
110
if(interval)
111
return;
112
113
start = new Date();
114
115
if(split) {
116
times.style.display = 'none';
117
times.innerHTML = '';
118
119
split = null;
120
}
121
122
function tick() {
123
var now = new Date();
124
125
if(split) {
126
var html = '<div class="total-time">'
127
+ renderTime(start, now)
128
+ '</div>'
129
+ '<div class="split-time">'
130
+ renderTime(split, now)
131
+ '</div>';
132
133
display.innerHTML = html;
134
} else {
135
var html = '<div class="total-time">'
136
+ renderTime(start, now)
137
+ '</div>';
138
139
display.innerHTML = html;
140
}
141
}
142
143
interval = setInterval(tick, 10); // once per 10 ms
144
}
145
146
function stopStopwatch() {
147
if(interval) {
148
clearInterval(interval);
149
150
interval = null;
151
}
152
}
153
154
function splitTime() {
155
if(interval) {
156
var now = new Date();
157
158
if (split == null) {
159
times.innerHTML += '<div class="split-time">'
160
+ renderTime(start, now)
161
+ '</div>';
162
163
times.style.display = 'block';
164
} else {
165
times.innerHTML += '<div class="split-time">'
166
+ renderTime(split, now)
167
+ '</div>';
168
}
169
170
split = now;
171
}
172
}
173
174
</script>
175
</body>
176
</html>