EN
React - import Google Fonts
0 points
In this article, we would like to show you how to use Google Fonts with React.
Quick solution:
1. Select Font and copy the <link>
element from the Use on the web section.
2. Paste the <link>
element into the <head>
section of the index.html file in the public/ folder.
3. Now you can use the font inside .css files in your app.
1. Go to the Google Fonts page, search and select the font. In this example, we select the Roboto font.
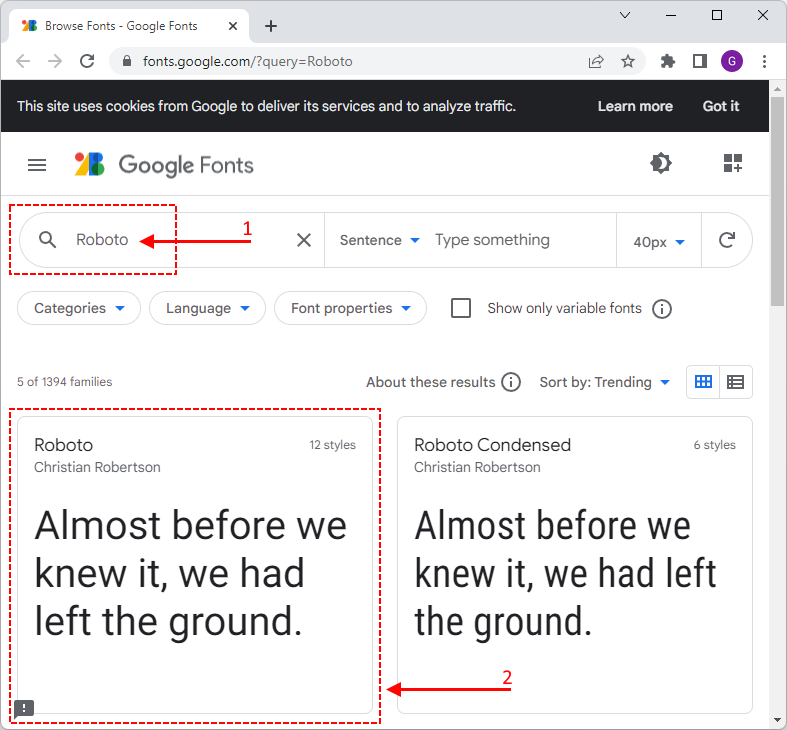
2. Select the font style and open View your selected families.
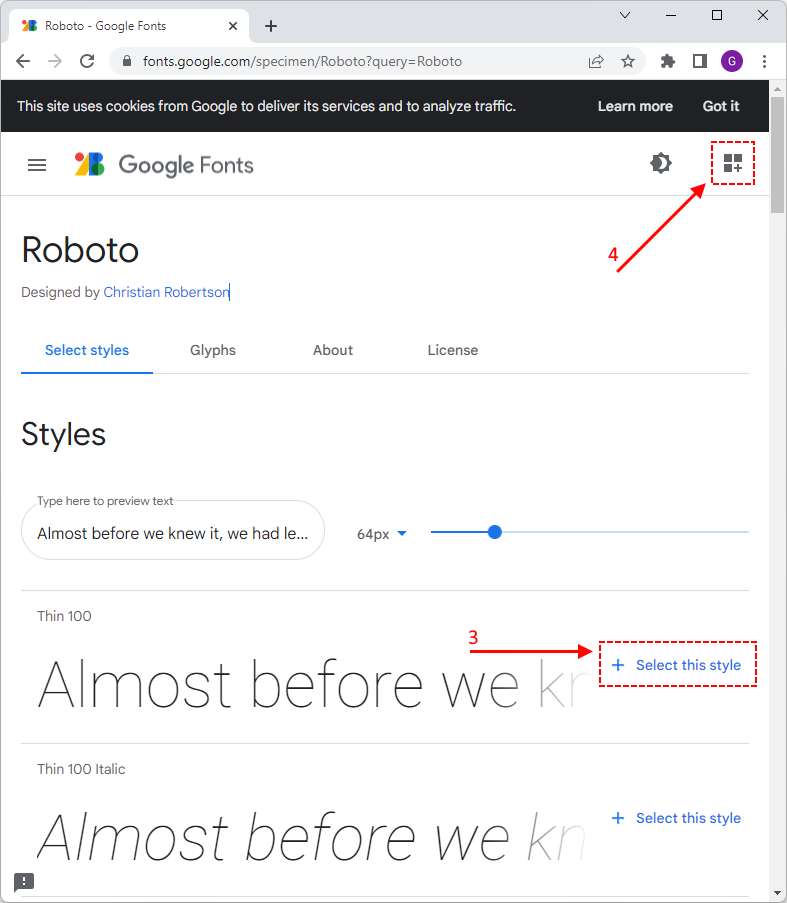
3. Select and copy the link
elements.
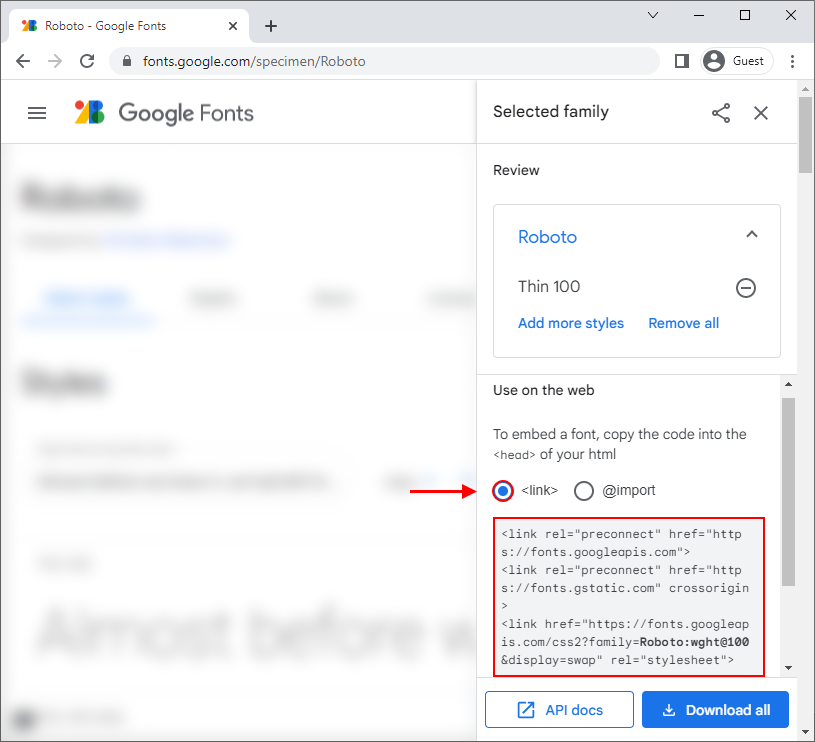
4. Paste link
elements to the <head>
of your index.html file.
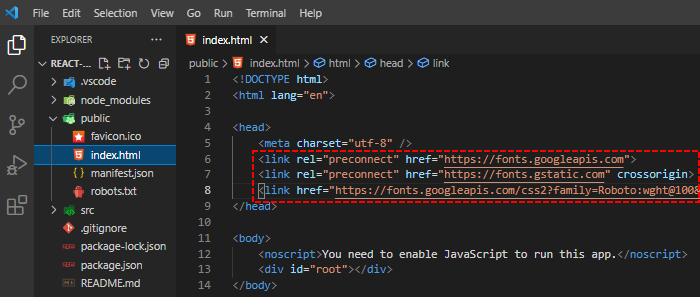
5. Assign the CSS rules into the element where you want to place the font.
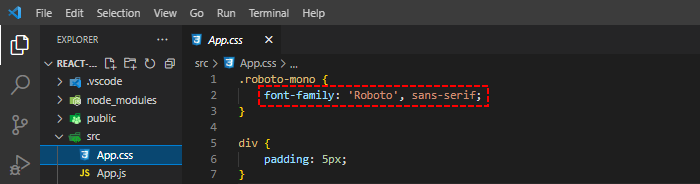
6. Import the CSS style into the .js file and run the app.
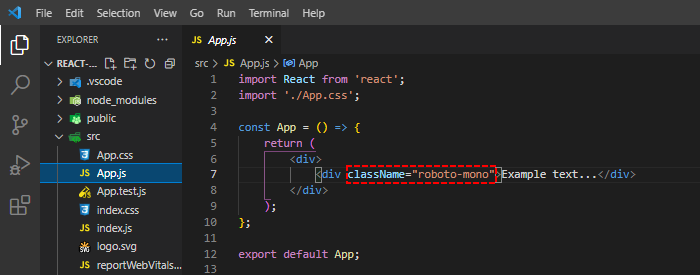
Result:
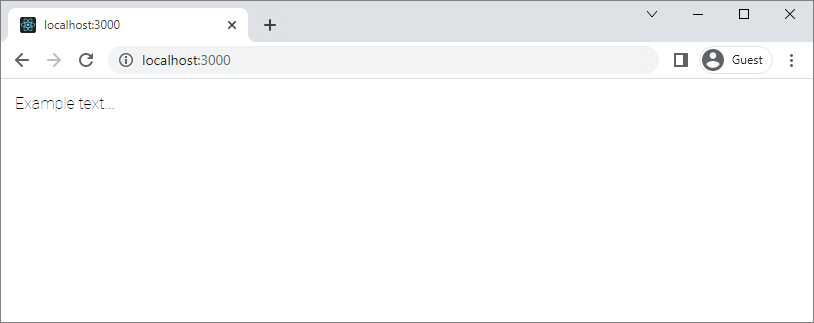
index.html
xxxxxxxxxx
1
2
3
<head>
4
<link rel="preconnect" href="https://fonts.googleapis.com">
5
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
6
<link href="https://fonts.googleapis.com/css2?family=Roboto:wght@100&display=swap" rel="stylesheet">
7
</head>
8
9
<body>
10
<div id="root"></div>
11
</body>
12
13
</html>
App.css
xxxxxxxxxx
1
.roboto-mono {
2
font-family: 'Roboto', sans-serif;
3
}
App.js
xxxxxxxxxx
1
import React from 'react';
2
import './App.css';
3
4
const App = () => {
5
return (
6
<div>
7
<div className="roboto-mono">Example text...</div>
8
</div>
9
);
10
};
11
12
export default App;