EN
JavaScript - get only certain part of canvas
0 points
In this article, we would like to show you how to get only a certain part of canvas using JavaScript
Quick solution:
xxxxxxxxxx
1
var canvas = document.querySelector('#canvas-id');
2
var context = canvas.getContext('2d');
3
context.drawImage(image, dx, dy);
or:
xxxxxxxxxx
1
var canvas = document.querySelector('#canvas-id');
2
var context = canvas.getContext('2d');
3
context.drawImage(image, sx, sy, sWidth, sHeight, dx, dy, dWidth, dHeight);
In this example, we use drawImage()
method with three arguments so we can get part of the canvas and place its upper-left corner into the dx
, dy
coordinates.
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
6
#my-canvas { border: 1px solid gray; }
7
8
</style>
9
</head>
10
<body>
11
<img id="image" src="https://dirask.com/static/bucket/1633539280748-ymAGm6LYq6--image.png" />
12
<br>
13
<canvas id="my-canvas" width="300" height="300"></canvas>
14
<script>
15
16
var canvas = document.querySelector('#my-canvas');
17
var context = canvas.getContext('2d');
18
19
var image = document.querySelector('#image');
20
21
image.onload = function() {
22
context.drawImage(image, 50, 100); // drawImage(image, dx, dy)
23
};
24
25
image.onerror = function() {
26
context.fillStyle = 'red';
27
context.font = '16px Arial';
28
context.fillText('Image loading error!', 10, 30);
29
};
30
31
</script>
32
</body>
33
</html>
Result:
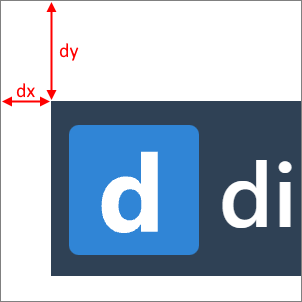
With this approach, we are able to specify the parameters of the part that we want to get, where we want to place it and its size on the destination canvas.
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
6
#my-canvas { border: 1px solid gray; }
7
8
</style>
9
</head>
10
<body>
11
<img id="image" src="https://dirask.com/static/bucket/1633539280748-ymAGm6LYq6--image.png" />
12
<br>
13
<canvas id="my-canvas" width="300" height="300"></canvas>
14
<script>
15
16
var canvas = document.querySelector('#my-canvas');
17
var context = canvas.getContext('2d');
18
19
var image = document.querySelector('#image'); // 440px x 175px
20
21
image.onload = function() {
22
context.drawImage(image, 20, 25, 110, 120, 50, 80, 110, 120);
23
};
24
25
image.onerror = function() {
26
context.fillStyle = 'red';
27
context.font = '16px Arial';
28
context.fillText('Image loading error!', 10, 30);
29
};
30
31
</script>
32
</body>
33
</html>
Result:
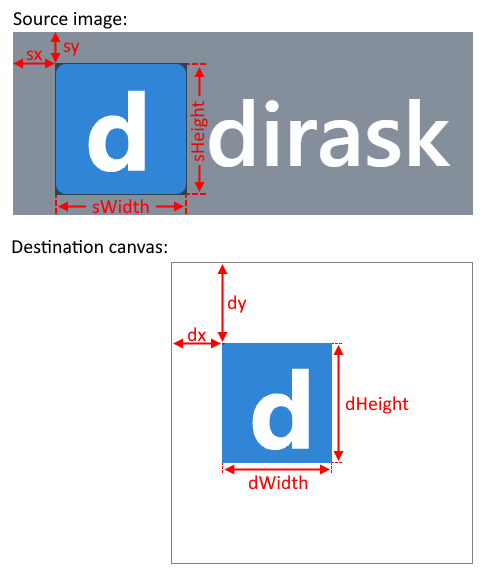