EN
JavaScript - set lineCap property on canvas
0
points
In this article, we would like to show you how to set lineCap
property while drawing on canvas using JavaScript.
Quick solution:
context.lineCap = 'round'; // 'square' or 'butt' (default)
// Usage example:
context.lineWidth = 20;
context.beginPath();
context.moveTo(10, 50);
context.lineTo(10, 100);
context.stroke();
drawLine();
Overview
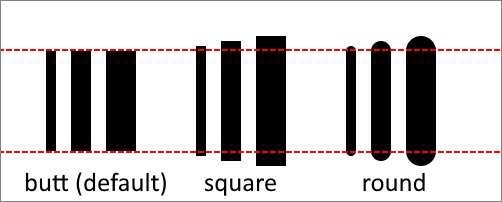
Practical example
In this example, we present three lines with different lineCap
property values.
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<style>
#my-canvas { border: 1px solid gray; }
</style>
</head>
<body>
<canvas id="my-canvas" width="200" height="200"></canvas>
<script>
var canvas = document.querySelector('#my-canvas');
var context = canvas.getContext('2d');
function drawLine() {
context.lineWidth = 20;
// line 1
context.lineCap = 'butt'; // <--- (default)
context.beginPath();
context.moveTo(50, 50);
context.lineTo(50, 150);
context.stroke();
// line 2
context.lineCap = 'square'; // <---
context.beginPath();
context.moveTo(100, 50);
context.lineTo(100, 150);
context.stroke();
// line 3
context.lineCap = 'round'; // <---
context.beginPath();
context.moveTo(150, 50);
context.lineTo(150, 150);
context.stroke();
}
drawLine();
</script>
</body>
</html>