EN
JavaScript - track last 10 site loading times and print statistics
4 points
This article contains logic that lets to print page loading times.
As page loading times, we understand the time necessary to load only site entry point (it means: index.html
or index.php
or ... - depending on technology).
Results presented are in the following way:
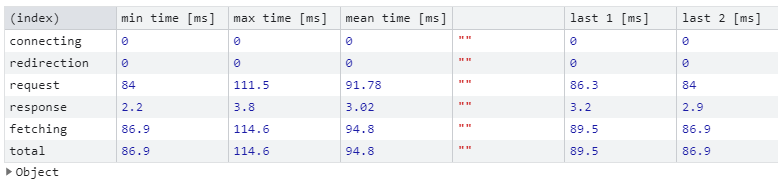
Usage:
- paste below script to
body
element, - open Console in the Google Chrome DevTools,
- refresh the page many times to collect loading times (refresh more than 10 times).
Hint: change
var historySize = 10;
to collect the different amounts of the last times.
Source code ():
xxxxxxxxxx
1
// storage logic
2
3
function readArray(name) {
4
var json = localStorage.getItem(name);
5
if (json) {
6
return JSON.parse(json);
7
}
8
return null;
9
}
10
11
function saveArray(name, array) {
12
var json = JSON.stringify(array);
13
localStorage.setItem(name, json);
14
}
15
16
// performance logic
17
18
function checkPerformance() {
19
if (performance.getEntries) {
20
var entries = performance.getEntries();
21
if (entries.length > 0) {
22
return entries[0];
23
}
24
}
25
return null;
26
}
27
28
function getTimes() {
29
var performance = checkPerformance();
30
if (performance) {
31
return {
32
connectingDuration: performance.connectEnd - performance.connectStart,
33
redirectionDuration: performance.redirectEnd - performance.redirectStart,
34
requestDuration: performance.responseStart - performance.requestStart,
35
responseDuration: performance.responseEnd - performance.responseStart,
36
fetchingDuration: performance.responseEnd - performance.requestStart, // request time + TTFB + response time (downloading)
37
totalDuration: performance.responseEnd - (performance.redirectStart || performance.connectStart)
38
};
39
}
40
return null;
41
}
42
43
// arrays logic
44
45
function createArray(count) {
46
var array = Array(count);
47
for (var i = 0; i < count; ++i) {
48
array[i] = 0;
49
}
50
return array;
51
}
52
53
function resizeArray(array, size) {
54
var changeSize = size - array.length;
55
if (changeSize > 0) {
56
return array.concat(createArray(changeSize));
57
} else {
58
return array.slice(0, size);
59
}
60
}
61
62
// math logic
63
64
function isLesser(result, value) {
65
return result < value;
66
}
67
68
function isBigger(result, value) {
69
return result > value;
70
}
71
72
function roundNumber(value, precision) {
73
var power = Math.pow(10, precision);
74
return Math.round(value * power) / power;
75
}
76
77
function calculateSum(array) {
78
var sum = 0;
79
for (var i = 0; i < array.length; ++i) {
80
sum += array[i];
81
}
82
return sum;
83
}
84
85
function calculateMean(array) {
86
var sum = calculateSum(array);
87
return sum / array.length;
88
}
89
90
function findValue(array, compare) {
91
if (array.length > 0) {
92
var result = array[0];
93
for (var i = 1; i < array.length; ++i) {
94
var value = array[i];
95
if (compare(value, result)) {
96
result = value;
97
}
98
}
99
return result;
100
}
101
return NaN;
102
}
103
104
// processing logic
105
106
function updateValues(historySize, parameterName, parameterValue) {
107
var storedValues = readArray(parameterName + 's');
108
109
if (storedValues == null) {
110
storedValues = createArray(historySize);
111
} else {
112
if (storedValues.length != historySize) {
113
storedValues = resizeArray(storedValues, historySize);
114
}
115
}
116
117
storedValues.shift();
118
storedValues.push(parameterValue);
119
120
saveArray(parameterName + 's', storedValues);
121
122
return storedValues;
123
}
124
125
// Usage example:
126
127
var historySize = 10;
128
129
window.addEventListener('load', function() {
130
var currentTimes = getTimes();
131
if (currentTimes) {
132
var gatheredTimes = {};
133
134
for (var parameterName in currentTimes) {
135
var storedValues = updateValues(historySize, parameterName, currentTimes[parameterName])
136
137
var minTime = findValue(storedValues, isLesser);
138
var maxTime = findValue(storedValues, isBigger);
139
var meanTime = calculateMean(storedValues);
140
141
var parameterTimes = {
142
'min time [ms]': roundNumber(minTime, 3),
143
'max time [ms]': roundNumber(maxTime, 3),
144
'mean time [ms]': roundNumber(meanTime, 3),
145
'': '' // used as separator
146
};
147
148
for (var i = 0; i < historySize; ++i) {
149
parameterTimes['last ' + (i + 1) + ' [ms]'] = roundNumber(storedValues[historySize - i - 1], 3);
150
}
151
152
gatheredTimes[parameterName.substring(0, parameterName.length - 8)] = parameterTimes;
153
}
154
155
console.table(gatheredTimes);
156
}
157
});