EN
JavaScript - measure page loading times (connection, redirection, fetching, request, response, total)
4 points
In this short article, we would like to focus on how to measure page loading times using JavaScript Performance API.
Presented below solution returns times similar to provided by Google Chrome DevTools.
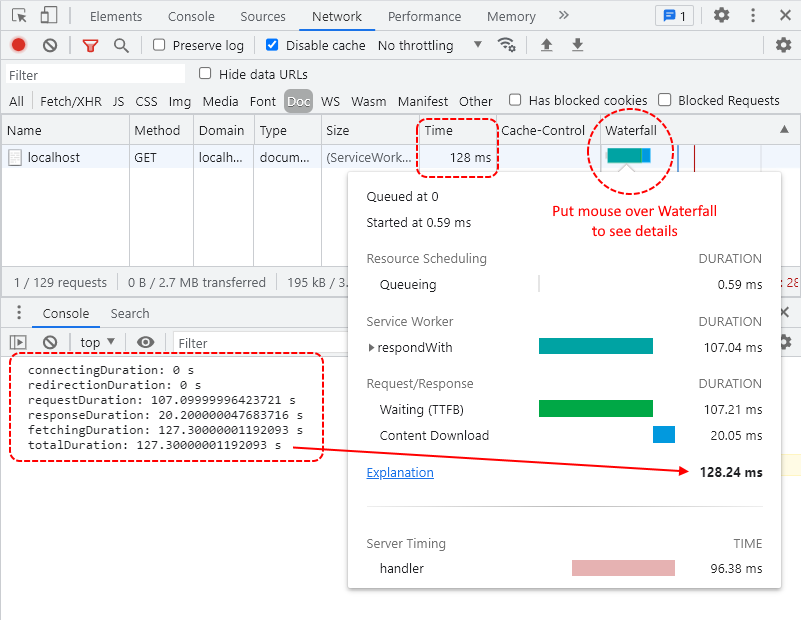
Note: copy the below
<script>
code and put it on your site.
Practical example:
xxxxxxxxxx
1
2
<html>
3
<head>
4
<script>
5
6
function checkPerformance() {
7
if (performance.getEntries) {
8
var entries = performance.getEntries();
9
if (entries.length > 0) {
10
return entries[0];
11
}
12
}
13
return null;
14
}
15
16
17
function getTimes() {
18
var performance = checkPerformance();
19
if (performance) {
20
return {
21
connectingDuration: performance.connectEnd - performance.connectStart,
22
redirectionDuration: performance.redirectEnd - performance.redirectStart,
23
requestDuration: performance.responseStart - performance.requestStart,
24
responseDuration: performance.responseEnd - performance.responseStart,
25
fetchingDuration: performance.responseEnd - performance.requestStart, // request time + TTFB + response time (downloading)
26
totalDuration: performance.responseEnd - (performance.redirectStart || performance.connectStart)
27
};
28
}
29
return null;
30
}
31
32
function onLoad() {
33
var times = getTimes();
34
if (times) {
35
console.log(
36
'connectingDuration: ' + times.connectingDuration + ' s\n' +
37
'redirectionDuration: ' + times.redirectionDuration + ' s\n' +
38
'requestDuration: ' + times.requestDuration + ' s\n' +
39
'responseDuration: ' + times.responseDuration + ' s\n' +
40
'fetchingDuration: ' + times.fetchingDuration + ' s\n' +
41
'totalDuration: ' + times.totalDuration + ' s'
42
);
43
}
44
}
45
46
window.addEventListener('load', onLoad); // it is good to get times after page is loaded
47
48
</script>
49
</head>
50
<body>
51
<p>Web page content...</p>
52
</body>
53
</html>